


How Can I Trigger Compile-Time Errors in Go for Variadic Functions Called with No Arguments?
Go: Triggering Compile-Time Errors with Custom Libraries
When designing custom library functions, it's essential to consider error handling, even for variadic functions. While calling a variadic function with no arguments is valid in Go, it can lead to bugs in the caller's code. This article explores the possibilities of triggering compile-time errors in such scenarios.
Variadic Functions and Empty Argument Lists
Variadic functions allow callers to pass a variable number of arguments. However, calling a variadic function with no arguments is not considered an error by the language specification. This can create a challenge for library designers who want to ensure that functions are used with appropriate arguments.
Signature Modification
One approach to enforce minimum arguments is to modify the function signature. Instead of a purely variadic parameter, a non-variadic parameter followed by a variadic parameter can be used. This ensures that at least one argument must be provided:
func min(first int, rest ...int) int { // ... Logic }
This function requires at least one argument. Attempting to call it without arguments will result in a compile-time error.
Custom Error Handling
If modifying the function signature is not feasible, custom error handling within the function can be implemented. However, this will only trigger runtime errors, not compile-time errors:
func min(args ...int) int { if len(args) == 0 { // Panic or exit the application } // ... Logic }
Balancing Flexibility and Error Prevention
The choice between enforcing minimum arguments at compile-time or handling it at runtime involves a trade-off between flexibility and error prevention. Signature modification ensures that errors are caught at compile-time, while runtime error handling allows for more flexible function usage but requires the caller to be responsible for passing the correct arguments.
Consider the specific requirements of the library and caller interface to determine the appropriate approach.
The above is the detailed content of How Can I Trigger Compile-Time Errors in Go for Variadic Functions Called with No Arguments?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


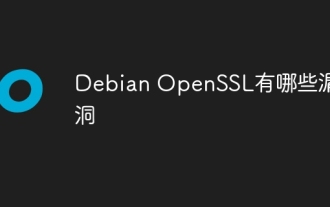
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
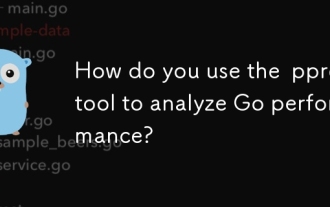
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
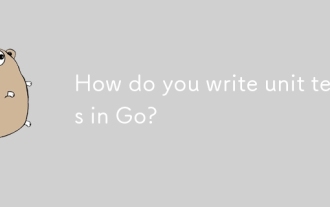
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
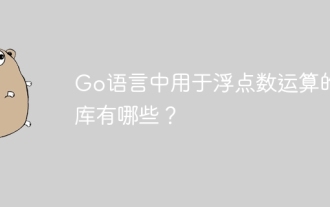
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
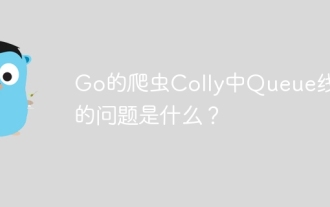
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
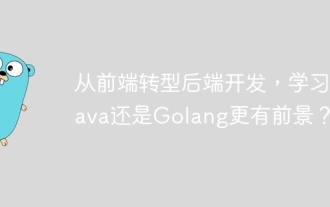
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
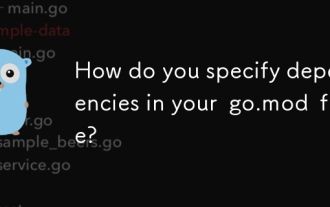
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
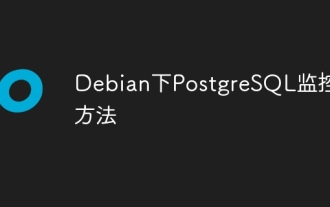
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
