How Can I Access Resource Paths Inside a Java JAR File?
Dec 18, 2024 am 12:07 AMRetrieving Resource Paths within Java JAR Files
In Java, accessing resources packaged within JAR files can be challenging. While streaming resource content is straightforward, obtaining an accessible file path can be elusive.
One approach attempts to create a File object using the getResource method:
ClassLoader classLoader = getClass().getClassLoader(); File file = new File(classLoader.getResource("config/netclient.p").getFile());
However, this often fails with a FileNotFoundException, as the resource may not have a corresponding file on the file system.
Understanding the Resource Concept
JAR files bundle resources in an archive, which may not be directly accessible as physical files. The classloader handles resource loading and can provide access via streams.
Alternative: Streaming Resource Contents
To access resource content without a file path, use:
ClassLoader classLoader = getClass().getClassLoader(); PrintInputStream(classLoader.getResourceAsStream("config/netclient.p"));
Temporary File Option
If a File object is indispensable, consider copying the stream into a temporary file:
InputStream resourceStream = classLoader.getResourceAsStream("config/netclient.p"); File tempFile = File.createTempFile("resource", ".tmp"); try (OutputStream outputStream = new FileOutputStream(tempFile)) { resourceStream.transferTo(outputStream); }
Once copied, you can manipulate the temporary file as needed.
The above is the detailed content of How Can I Access Resource Paths Inside a Java JAR File?. For more information, please follow other related articles on the PHP Chinese website!

Hot tools Tags

Hot Article

Hot tools Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
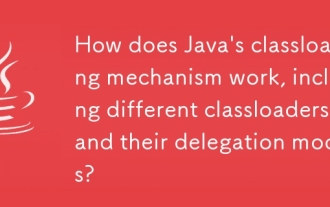
How does Java's classloading mechanism work, including different classloaders and their delegation models?
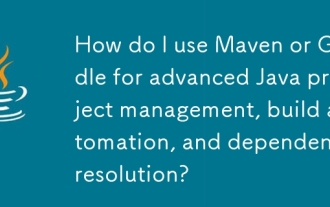
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
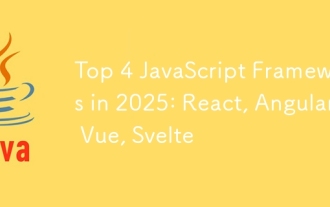
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
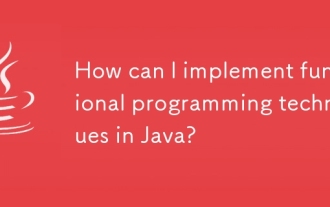
How can I implement functional programming techniques in Java?
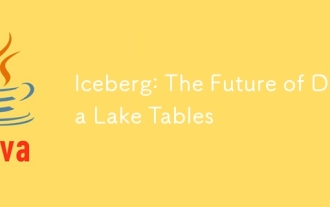
Iceberg: The Future of Data Lake Tables
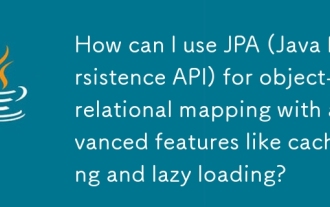
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
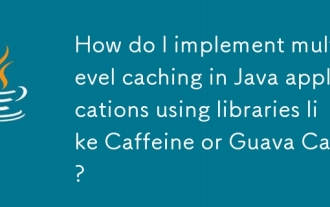
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
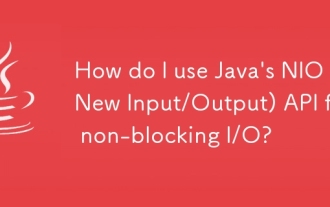
How do I use Java's NIO (New Input/Output) API for non-blocking I/O?
