How to Optimize SQL Queries for Tables with Comma-Separated Values?
Optimizing SQL Queries for Tables with Comma-Separated Column Values
When dealing with tables where a column contains comma-separated values, executing specific queries can be challenging. A common scenario is retrieving all related data when searching for specific values within the comma-separated column.
Issue:
You need to retrieve all usernames associated with a particular value in a comma-separated column. For instance, from the table structure below, you want to find all usernames when you search for "new1."
Tables:
CREATE TABLE tblA ( id int NOT NULL AUTO_INCREMENT , user varchar(255), category int(255), PRIMARY KEY (id) ); CREATE TABLE tblB ( id int NOT NULL AUTO_INCREMENT , username varchar(255), userid int(255), PRIMARY KEY (id) ); CREATE TABLE tblC ( id int NOT NULL AUTO_INCREMENT , nname varchar(255), userids varchar(255), PRIMARY KEY (id) ); INSERT INTO tblA (user, category ) VALUES ('1', '1'), ('1', '2'), ('1', '3'), ('1', '1'), ('2', '1'), ('2', '1'), ('2', '1'), ('2', '1'), ('3', '1'), ('2', '1'), ('4', '1'), ('4', '1'), ('2', '1'); INSERT INTO tblB (userid, username ) VALUES ('1', 'A'), ('2', 'B'), ('3', 'C'), ('4', 'D'), ('5', 'E'); INSERT INTO tblC (id, nname,userids ) VALUES ('1', 'new1','1,2'), ('2', 'new2','1,3'), ('3', 'new3','1,4'), ('4', 'new4','3,2'), ('5', 'new5','5,2');
Query:
select * where nname="new1" from tblC CROSS JOIN tblB ON tblB.userid=(SELECT userids FROM substr(tblC.userids,','))
Limitations:
This query relies on the SUBSTR and FIND_IN_SET functions, which can be inefficient for large datasets. Moreover, it assumes only one comma-separated value per row, which may not always be the case.
Recommended Solution:
Database Normalization:
Normalize your database schema by creating a separate table for the comma-separated values. This eliminates the inefficiency of searching through strings and simplifies querying.
Example Schema:
CREATE TABLE tblC ( id int NOT NULL AUTO_INCREMENT , nname varchar(255), PRIMARY KEY (id) ); CREATE TABLE tblC_user ( c_id int NOT NULL, userid int NOT NULL ); INSERT INTO tblC (id, nname) VALUES ('1', 'new1'), ('2', 'new2'), ('3', 'new3'), ('4', 'new4'), ('5', 'new5'); INSERT INTO tblC_user (c_id, userid) VALUES ('1','1'), ('1','2'), ('2','1'), ('2','3'), ('3','1'), ('3','4'), ('4','3'), ('4','2'), ('5','5'), ('5','2');
Optimized Query:
select * from tblC c join tblC_user cu on(c.id = cu.c_id) join tblB b on (b.userid = cu.userid) where c.nname="new1"
Benefits:
- Improved performance due to efficient join operations
- Reduced complexity by eliminating string manipulation functions
- Maintainability and flexibility in accommodating future changes to the data structure
The above is the detailed content of How to Optimize SQL Queries for Tables with Comma-Separated Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










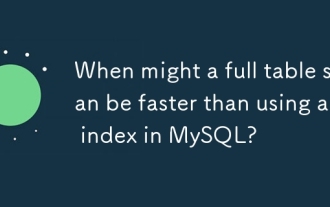
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
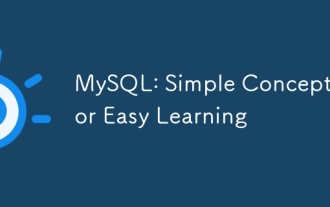
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
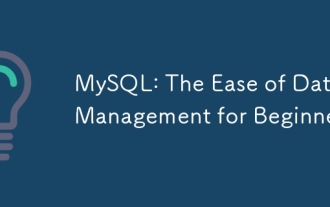
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
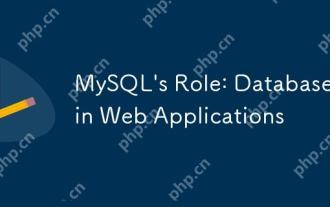
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
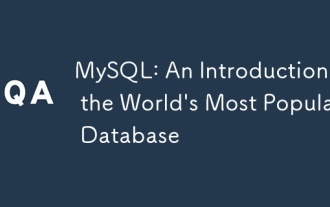
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
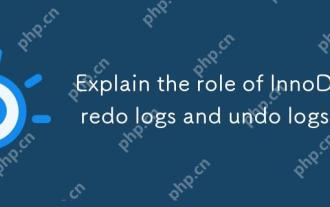
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
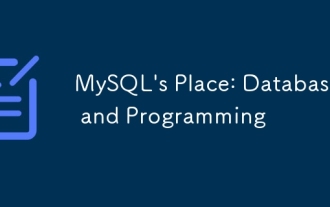
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
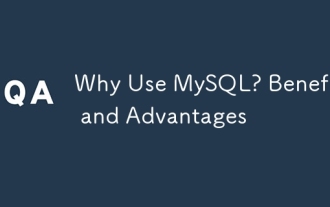
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
