


How Does JavaScript Handle Pass-by-Value and Pass-by-Reference with Primitive Types and Objects?
JavaScript Pass-by-Value vs. Pass-by-Reference
In JavaScript, all variables are passed by value, which means that a copy of the original value is made and passed to the function. However, when the value is an object, such as an array or an object literal, the copy is a reference to the original object.
Impact on Function Arguments
- Primitive: When a primitive (e.g., number, string) is passed to a function, any changes made to that value within the function are limited to the local scope of the function and do not affect the original variable outside the function.
- Object Reference: When an object reference is passed to a function, changes made to the object's properties within the function are reflected in the original object, even outside the function.
Impact on Variables Outside Functions
- Pass-by-Reference: Object references are passed by reference, meaning that changes made to the object's properties within a function will affect the original object's properties, but any changes made to the reference itself (e.g., reassignment) within the function do not affect the original variable.
- Pass-by-Value: Primitive values and object references passed by value do not affect the original variable outside the function.
Examples
function f(a, b, c) { a = 3; // Reassignment changes the local variable only. b.push("foo"); // Property change affects the original object. c.first = false; // Property change affects the original object. } const x = 4; let y = ["eeny", "miny", "mo"]; let z = { first: true }; f(x, y, z); console.log(x, y, z.first); // Output: 4, ["eeny", "miny", "mo", "foo"], false
In the example above, the changes to the b and c objects are reflected in the original objects, while the reassignment of a has no effect.
In-depth Examples:
function f() { const a = ["1", "2", { foo: "bar" }]; const b = a[1]; // Copy the reference to the original array element a[1] = "4"; // Change the value in the original array console.log(b); // Output: "2" (Original value of the copied reference) }
In the first example, even though a has been modified, b still holds the original value because it is a copy of the reference.
function f() { const a = [{ yellow: "blue" }, { red: "cyan" }, { green: "magenta" }]; const b = a[1]; // Copy the reference to the original object a[1].red = "tan"; // Change the property in the original object console.log(b.red); // Output: "tan" (Property change is reflected in both variables) b.red = "black"; // Change the property through the reference console.log(a[1].red); // Output: "black" (Property change is reflected in both variables) }
In the second example, the change to a[1].red affects both a and b because they share the same object reference.
Creating Independent Copies
To create a fully independent copy of an object, you can use the JSON.parse() and JSON.stringify() methods to deserialize and serialize the object respectively. For example:
const originalObject = { foo: "bar" }; const independentCopy = JSON.parse(JSON.stringify(originalObject));
The above is the detailed content of How Does JavaScript Handle Pass-by-Value and Pass-by-Reference with Primitive Types and Objects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










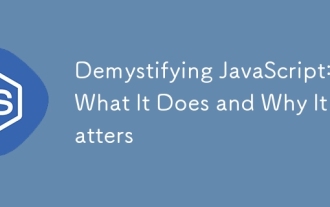
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
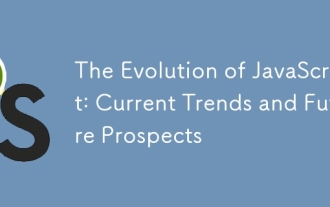
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
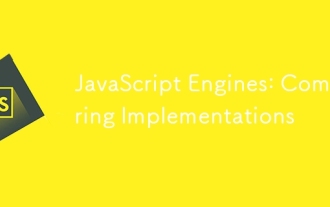
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
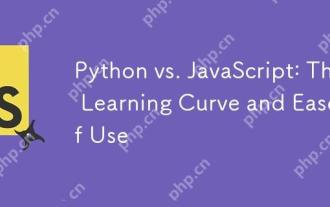
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
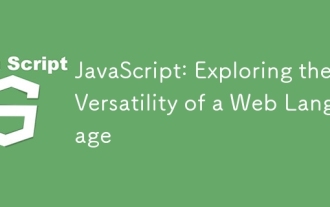
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
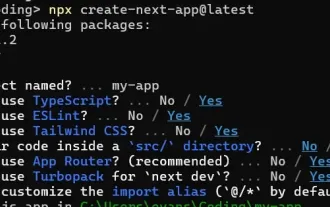
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
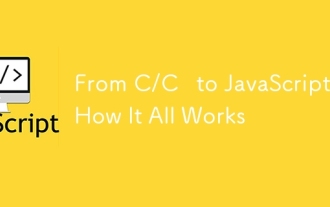
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
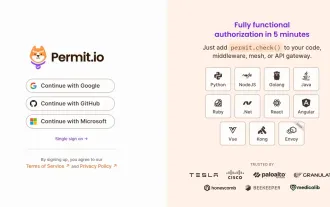
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
