How to Implement Partial Content Serving in Go HTTP Responses?
Serving Partial Content in HTTP Responses with Go
Serving partial content in HTTP responses allows clients to request specific ranges of a file. This is useful for handling media playback, where clients may only need to download portions of the file for seeking or looping purposes.
How to Serve Partial Content
To serve partial content using the http package in Go, you can use the ServeContent or ServeFile functions.
-
Using ServeFile: If you're serving files directly from the filesystem, you can use http.ServeFile:
http.HandleFunc("/file", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "my-file.mp3") })
Copy after login -
Using ServeContent: If you're serving content from a buffer or a stream, you can use http.ServeContent:
func handleContent(w http.ResponseWriter, r *http.Request) { content := getMyContent() http.ServeContent(w, r, "my-file.mp3", time.Now(), bytes.NewReader(content)) }
Copy after login
Both ServeFile and ServeContent handle range requests and set the appropriate content headers for partial content.
Implementing Partial Content Yourself
While the http package provides easy-to-use functions for serving partial content, you may need to implement it yourself in certain scenarios. Here's a brief overview:
- Handle range requests in your response handler.
- If a range is specified, seek to the requested offset in your content source.
- Read the requested bytes from your content source and write them to the response writer.
Implementing partial content serving yourself requires careful handling of status codes, request headers, and seeking in the content source.
Byte Serving and io.ReadSeeker
Byte serving is the underlying mechanism for serving partial content. It allows clients to request specific ranges of a file. To implement byte serving, your content must be represented as an io.ReadSeeker, an interface that provides methods for reading and seeking within a stream.
You can use the bytes.Reader type to represent your content as an io.ReadSeeker. The bytes.Reader can be initialized from a slice of bytes or any io.Reader implementation.
By creating an io.ReadSeeker view of your content, you can control how and when data is read from the content source, enabling you to handle range requests and serve partial content effectively.
The above is the detailed content of How to Implement Partial Content Serving in Go HTTP Responses?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










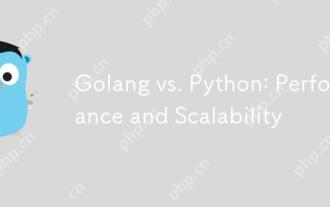
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
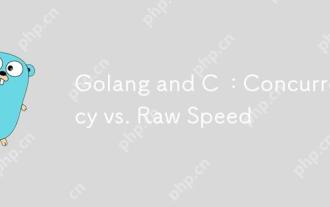
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
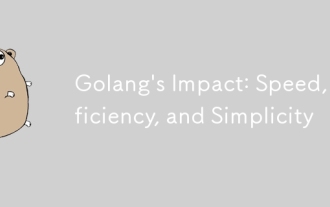
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
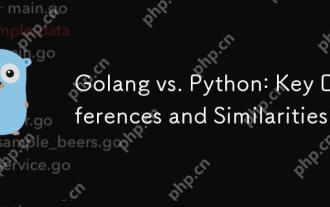
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
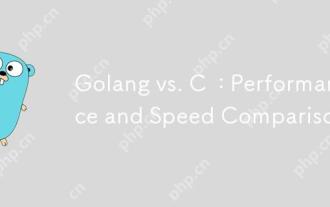
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
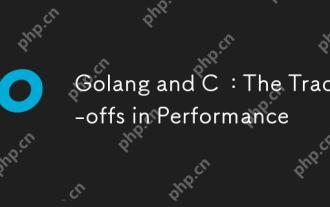
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
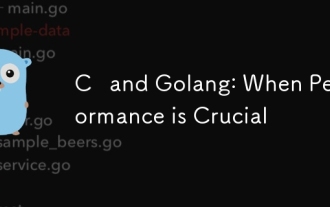
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
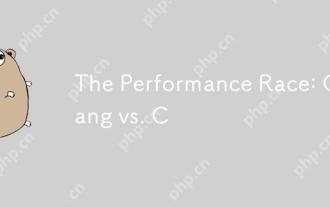
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
