


Why Does Redefining `str` Cause a `TypeError` in Subsequent Python Function Calls?
Redefining str Causes TypeError in Second Execution
The provided Python code exhibits peculiar behavior, where the call to example(1) executes without issue, but example(2) triggers a TypeError. This puzzling phenomenon stems from the problematic code sequence:
global str str = str(parameter)
Within this block, the code assigns the value str(parameter) to the global str variable. This redefinition essentially overrides the built-in Python name of the string type, causing conflicts with subsequent operations.
The Problem
The built-in str() function is used to convert objects into strings. By redefining str with str(parameter), the code effectively replaces the original function with a custom variable holding the converted value of parameter.
As a result, in the second call to example(2), when the code attempts to use str again, it's no longer a function but instead a string object. Consequently, the interpreter raises a TypeError because string objects cannot be called like functions.
The Solution
To rectify this issue, refrain from modifying the built-in str name. Instead, assign a different name to the local variable that stores the converted value. Additionally, remove the global statement, which is unnecessary since the local variable is not modifying any existing global variable.
Here's the corrected code:
def example(parameter): s = str(parameter) # Use a different name for the local variable print(s) # Use the newly created local variable example(1) example(2)
The above is the detailed content of Why Does Redefining `str` Cause a `TypeError` in Subsequent Python Function Calls?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










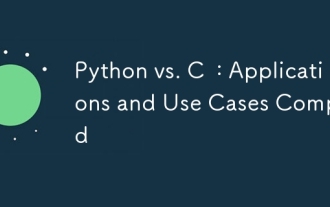
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
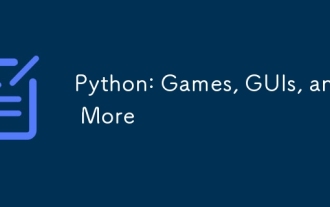
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
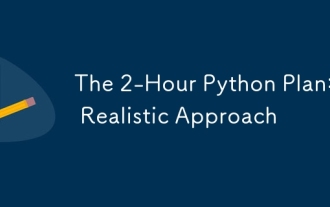
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
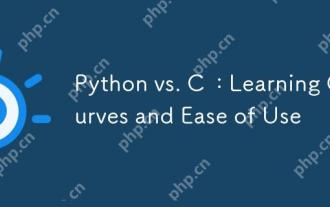
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
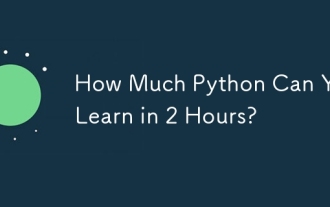
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
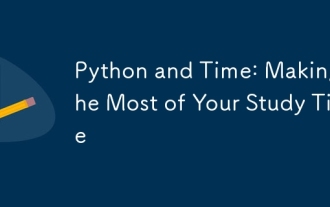
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
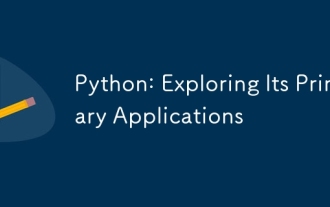
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
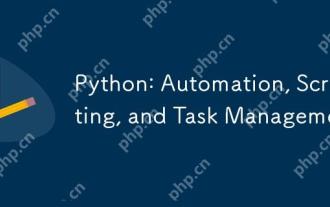
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
