


How Can I Gracefully Handle Ctrl C Signals in Go for Clean Program Termination?
Capturing Ctrl C for Cleanup Operations: A "Defer" Approach
Handling signals in Go allows developers to respond to specific events, including the termination of a program. One common scenario is capturing the Ctrl C (SIGINT) signal to perform any necessary cleanup operations before the program exits.
Creating a Channel for Signal Handling
To capture the SIGINT signal, we utilize the os/signal package. First, we create a channel to receive signals:
c := make(chan os.Signal, 1)
Subscribing to the Ctrl C Signal
Next, we notify the channel to listen for the SIGINT signal:
signal.Notify(c, os.Interrupt)
Initiating a Goroutine for Signal Handling
We create a goroutine to handle signals continuously:
go func(){ for sig := range c { // sig is a ^C, handle it } }()
Performing Cleanup Operations
Within the goroutine, we can define the cleanup operations to be performed when Ctrl C is pressed. This could include writing data to disk, saving preferences, or any other task that should be completed before the program exits.
The manner in which you cause your program to terminate and print information based on the Ctrl C input is flexible and depends on the specific requirements of your program.
The above is the detailed content of How Can I Gracefully Handle Ctrl C Signals in Go for Clean Program Termination?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
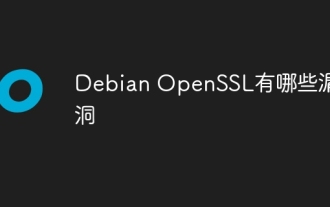
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
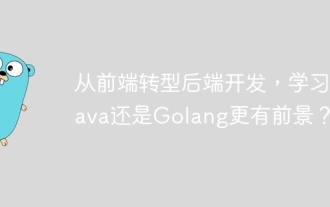
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
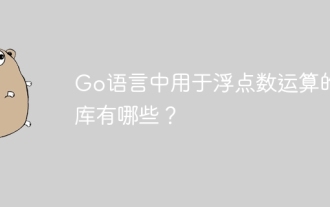
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
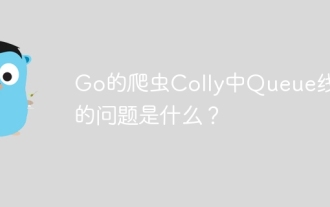
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
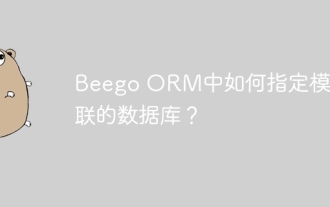
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
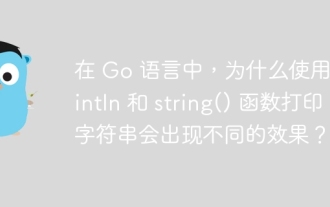
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
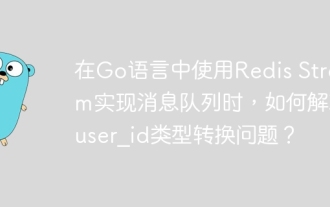
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
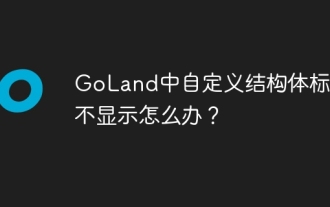
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
