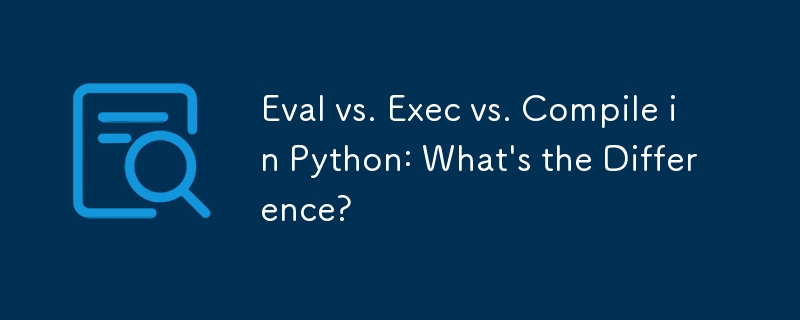
Eval, Exec, and Compile: A Comparative Analysis
Differences in Functionality
Eval evaluates a single expression dynamically and returns the result.
Exec executes a given block of code and discards its return value, primarily used for its side effects.
Compile and Modes
Compile plays a crucial role in both eval and exec:
-
'eval' mode: Compiles a single expression into bytecode that returns the evaluated value.
-
'exec' mode: Compiles code fragments of any type into bytecode that returns None (in Python 2, it was a statement and did not return anything).
Syntax and Differences
Python 2
- Exec is a statement.
- Eval is a function.
- Exec accepts a tuple of length 2 or 3 to define globals and locals (a hack for backward compatibility).
Python 3
- Exec is a function.
- Exec and eval behave identically when passed a code object.
Detailed Comparison
Evaluating Expressions:
- Exec does not accept expressions directly.
- Eval accepts only single expressions as strings or code objects.
Side Effects:
- Exec is used solely for side effects like variable modifications or function calls.
- Eval has no side effects, returning the value of the evaluated expression.
Statements and Code Blocks:
- Exec accepts code blocks containing statements, loops, and imports.
- Eval cannot handle statements directly; it requires compiled code objects for statements.
Example Usage
Calculating and Printing:
a = 5
result = eval('37 + a') # Eval calculates the expression and returns the result (42)
exec('print(37 + a)') # Exec executes the code (prints 42)
Copy after login
Modifying Variables:
a = 2
exec('a = 47') # Exec modifies the global variable `a`
result = eval('a = 47') # Eval throws an error because it cannot handle statements
Copy after login
The above is the detailed content of Eval vs. Exec vs. Compile in Python: What's the Difference?. For more information, please follow other related articles on the PHP Chinese website!