


How Can I Generate All Possible Combinations of a List's Elements in Python?
Generating All Possible Combinations of a List's Elements
You're seeking a method to generate all possible 2^N combinations from a list of 15 elements, accommodating combinations of any length while maintaining the original order. While your approach involving binary representation is a viable option, let's explore a more comprehensive solution.
Instead of relying on the binary representation, consider employing the itertools.combinations() function from the Python standard library. This function generates a sequence of all combinations of a given length from a given iterable. By varying the length parameter, you can obtain combinations of any desired size.
The following Python code demonstrates this approach:
1 2 3 4 5 6 7 8 9 |
|
This code will generate all 32,768 possible combinations of the elements in the stuff list, regardless of their length.
Alternatively, for a more streamlined solution, you can use the chain() and combinations() functions to generate a single sequence containing all combinations of all possible lengths:
1 2 3 4 5 6 7 8 9 |
|
This code achieves the same result but in a more concise and arguably elegant manner.
The above is the detailed content of How Can I Generate All Possible Combinations of a List's Elements in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
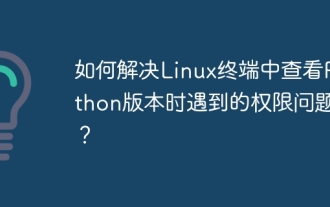
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
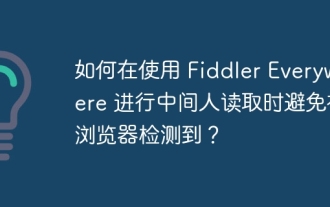
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
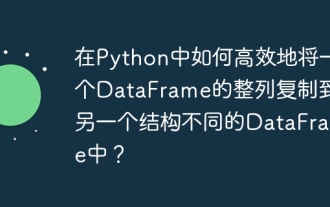
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
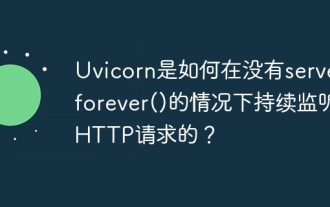
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
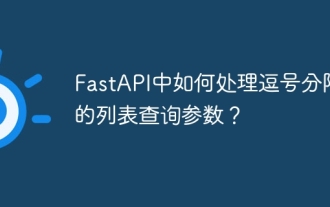
Fastapi ...
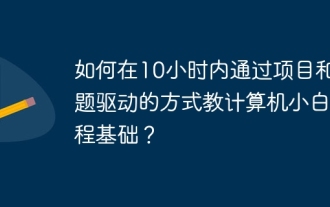
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
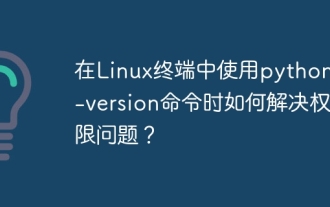
Using python in Linux terminal...
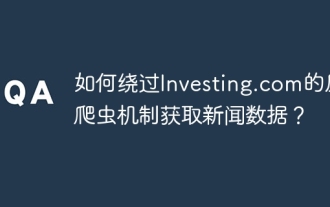
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
