How Can I Save and Restore Trained TensorFlow Models?
Saving and Restoring Trained TensorFlow Models
TensorFlow provides seamless capabilities for saving and restoring trained models, allowing you to persist and reuse your models in various scenarios.
Saving the Model
To save a trained model in TensorFlow, you can use the tf.train.Saver class. Here's an example:
import tensorflow as tf # Prepare placeholders and variables w1 = tf.placeholder(tf.float32, name="w1") w2 = tf.placeholder(tf.float32, name="w2") b1 = tf.Variable(2.0, name="bias") feed_dict = {w1: 4, w2: 8} # Define an operation to be restored w3 = tf.add(w1, w2) w4 = tf.multiply(w3, b1, name="op_to_restore") sess = tf.Session() sess.run(tf.global_variables_initializer()) # Create a saver object saver = tf.train.Saver() # Run the operation and save the graph print(sess.run(w4, feed_dict)) saver.save(sess, 'my_test_model', global_step=1000)
Restoring the Model
To restore a previously saved model, you can use the following process:
import tensorflow as tf sess = tf.Session() # Load the meta graph and restore weights saver = tf.train.import_meta_graph('my_test_model-1000.meta') saver.restore(sess, tf.train.latest_checkpoint('./')) # Access saved variables directly print(sess.run('bias:0')) # Prints 2 (the bias value) # Access and create feed-dict for new input data graph = tf.get_default_graph() w1 = graph.get_tensor_by_name("w1:0") w2 = graph.get_tensor_by_name("w2:0") feed_dict = {w1: 13.0, w2: 17.0} # Access the desired operation op_to_restore = graph.get_tensor_by_name("op_to_restore:0") print(sess.run(op_to_restore, feed_dict)) # Prints 60 ((w1 + w2) * b1)
For additional scenarios and use cases, refer to the resources provided in the provided answers, which delve deeper into saving and restoring TensorFlow models.
The above is the detailed content of How Can I Save and Restore Trained TensorFlow Models?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










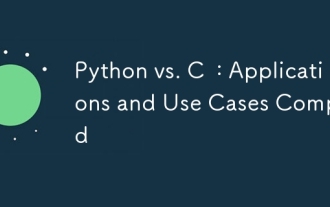
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
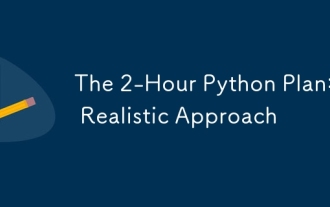
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
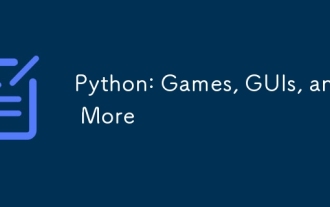
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
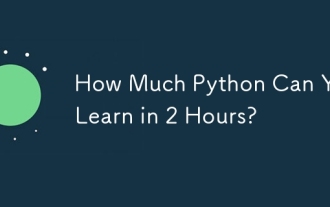
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
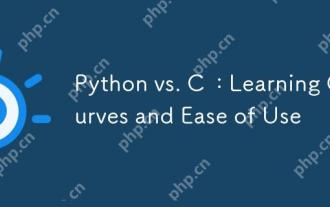
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
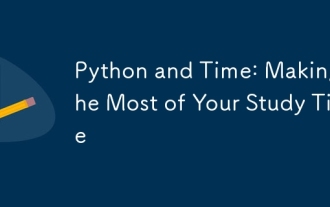
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
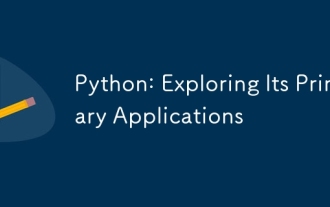
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
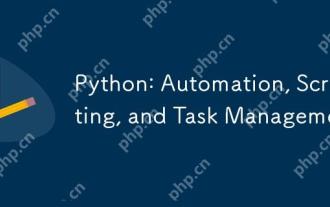
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
