


How to Fix the 'Path to Driver Executable' Error When Using Selenium with Firefox?
Selenium Using Java: Resolving the "Path to Driver Executable" Issue
When attempting to launch Mozilla using Selenium, it's common to encounter the error: "The path to the driver executable must be set by the webdriver.gecko.driver system property." This issue arises because Selenium requires the location of the "geckodriver" executable to be set so it can communicate with the browser.
Solution
The solution involves ensuring that the system path includes the directory where the "geckodriver" executable is located:
- Unix Systems: Add the directory to the system path using the following command (bash-compatible shell):
export PATH=$PATH:/path/to/geckodriver
- Windows Systems: Update the Path system variable to include the full directory path to the executable.
Configuring Selenium for Marionette
To use Marionette, an automation driver for Firefox, update your desired capabilities:
Java
Set the system property to the geckodriver location and initialize the driver using desired capabilities:
System.setProperty("webdriver.gecko.driver", "path/to/geckodriver.exe"); DesiredCapabilities capabilities = DesiredCapabilities.firefox(); capabilities.setCapability("marionette", true); WebDriver driver = new MarionetteDriver(capabilities);
Selenium 3
Simply use the default FirefoxDriver without any additional configurations.
.NET
Use the FirefoxOptions class:
var driver = new FirefoxDriver(new FirefoxOptions());
Python
Import the required modules and set the capabilities:
from selenium import webdriver from selenium.webdriver.common.desired_capabilities import DesiredCapabilities caps = DesiredCapabilities.FIREFOX caps["marionette"] = True driver = webdriver.Firefox(capabilities=caps)
Ruby
Set the path for Firefox and enable Marionette:
require 'selenium-webdriver' Selenium::WebDriver::Firefox::Binary.path = "/path/to/firefox" driver = Selenium::WebDriver.for :firefox, marionette: true
JavaScript (Node.js)
Set the capabilities for Marionette:
const webdriver = require('selenium-webdriver'); const Capabilities = require('selenium-webdriver/lib/capabilities').Capabilities; var capabilities = Capabilities.firefox(); capabilities.set('marionette', true); var driver = new webdriver.Builder().withCapabilities(capabilities).build();
Additionally, for RemoteWebDriver usage in any language:
Python
Set the desired capabilities:
caps = DesiredCapabilities.FIREFOX caps["marionette"] = True driver = webdriver.Firefox(capabilities=caps)
Ruby
Use the Capabilities class:
caps = Selenium::WebDriver::Remote::Capabilities.firefox marionette: true, firefox_binary: "/path/to/firefox" driver = Selenium::WebDriver.for :remote, desired_capabilities: caps
Java
DesiredCapabilities capabilities = DesiredCapabilities.firefox(); capabilities.setCapability("marionette", true); WebDriver driver = new RemoteWebDriver(capabilities);
.NET
DesiredCapabilities capabilities = DesiredCapabilities.Firefox(); capabilities.SetCapability("marionette", true); var driver = new RemoteWebDriver(capabilities);
By following these steps, you can resolve the "path to driver executable" issue and successfully launch Mozilla using Selenium.
The above is the detailed content of How to Fix the 'Path to Driver Executable' Error When Using Selenium with Firefox?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










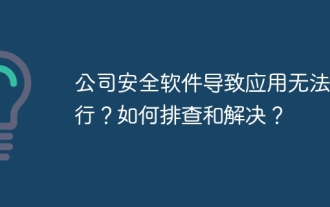
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
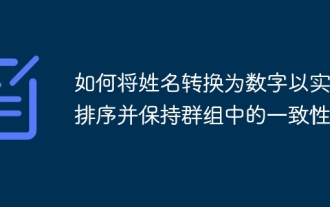
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
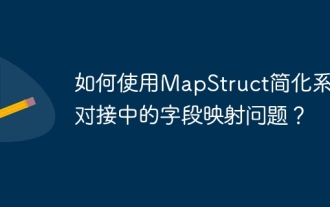
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
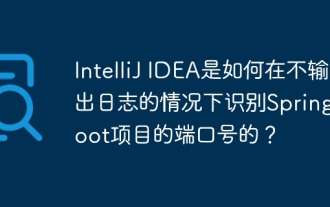
Start Spring using IntelliJIDEAUltimate version...
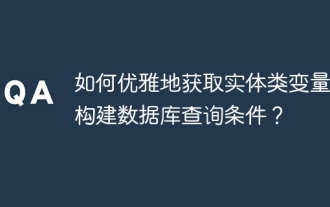
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
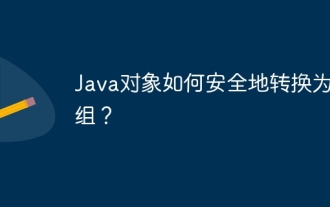
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
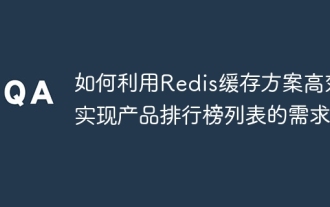
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
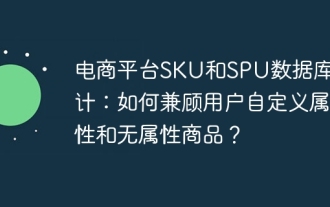
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
