How to Implement Java 8's Equivalent of Java 9's `takeWhile` for Streams?
Java 8 Stream: Limiting by Predicate
In scenarios with potentially infinite streams, we often encounter the need to limit the stream until the first element fails a specific predicate. Java 9 introduced the useful takeWhile operation, enabling this functionality effortlessly.
Unfortunately, Java 8 lacks such an operation. Thus, we must explore alternative approaches to achieve this behavior.
Implementing takeWhile in Java 8
To implement takeWhile in Java 8, we can leverage the following strategy:
- Create an iterator from the stream using iterator().
- Iterate through the iterator until the predicate fails.
- Collect the filtered elements into a new stream using generate().
Below is an example implementation:
import java.util.Iterator; import java.util.stream.Stream; public class StreamTakeWhile { public static <T> Stream<T> takeWhile(Stream<T> stream, java.util.function.Predicate<T> predicate) { Iterator<T> iterator = stream.iterator(); return Stream.generate(() -> { if (iterator.hasNext() && predicate.test(iterator.next())) { return iterator.next(); } return null; }); } public static void main(String[] args) { StreamTakeWhile.takeWhile(Stream.iterate(1, n -> n + 1), n -> n < 10) .forEach(System.out::println); } }
Using Java 9 takeWhile
With Java 9, you can directly utilize the takeWhile operation as shown below:
IntStream .iterate(1, n -> n + 1) .takeWhile(n -> n < 10) .forEach(System.out::println);
The above is the detailed content of How to Implement Java 8's Equivalent of Java 9's `takeWhile` for Streams?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










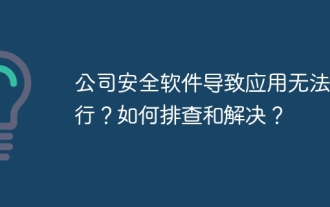
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
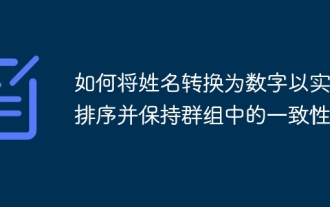
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
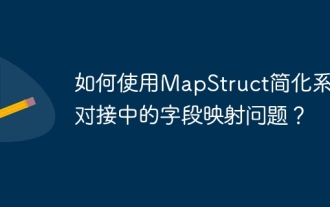
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
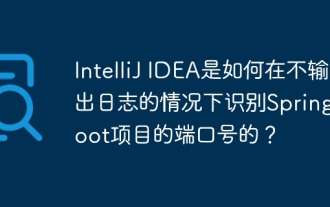
Start Spring using IntelliJIDEAUltimate version...
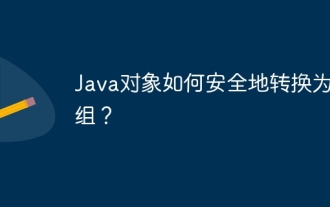
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
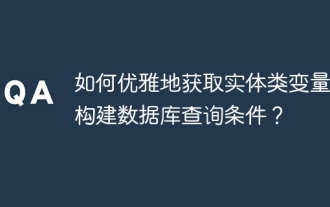
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
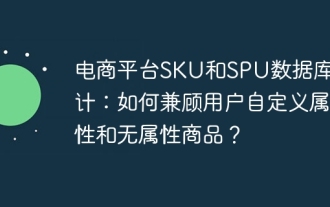
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
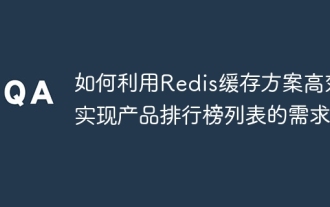
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
