


How to Efficiently Parse Complex JSON Structures in Go Using `json.Unmarshal`?
Parsing Complex JSON with Go's Unmarshal
The encoding/json package provides the json.Unmarshal function for parsing JSON data. This function can be used to unmarshal a JSON string into a predefined struct or an interface{} value.
When dealing with complex JSON structures that may include nested arrays and objects, using interface{} allows developers to handle unforeseen data structures. For instance, consider the following JSON:
{ "k1": "v1", "k2": "v2", "k3": 10, "result": [ [ ["v4", "v5", {"k11": "v11", "k22": "v22"}], ... ], "v3" ] }
To parse this JSON, we can use the following code snippet:
import ( "encoding/json" "fmt" ) func main() { b := []byte(`{ "k1" : "v1", "k2" : "v2", "k3" : 10, "result" : [ [ ["v4", v5, {"k11" : "v11", "k22" : "v22"}] , ... , ["v4", v5, {"k33" : "v33", "k44" : "v44"} ] ], "v3" ] }`) var f interface{} err := json.Unmarshal(b, &f) if err != nil { fmt.Println("Error:", err) } // Access the data in the interface{} value m := f.(map[string]interface{}) fmt.Println("k1:", m["k1"]) fmt.Println("k3:", m["k3"]) for _, v := range m["result"].([]interface{}) { fmt.Println("Element:", v) } }
By iterating through the map and using a type switch, we can access the values with the correct types. This approach allows us to work with unknown JSON structures in a flexible and type-safe manner.
The above is the detailed content of How to Efficiently Parse Complex JSON Structures in Go Using `json.Unmarshal`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
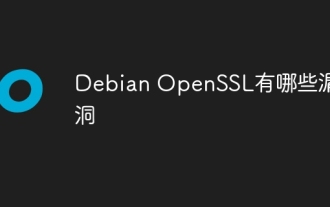
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
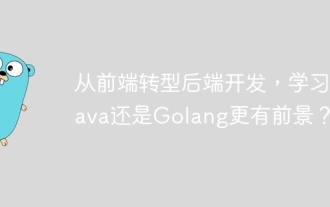
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
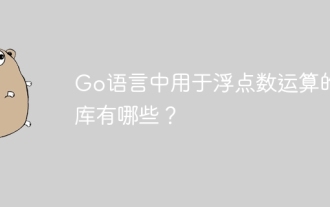
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
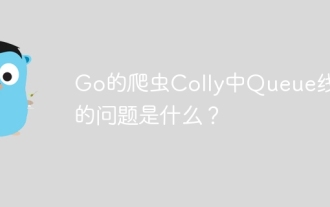
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
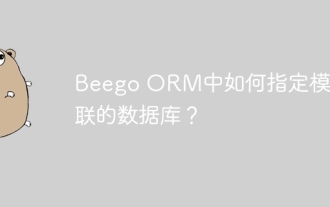
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
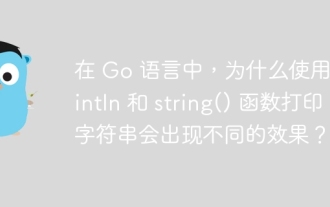
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
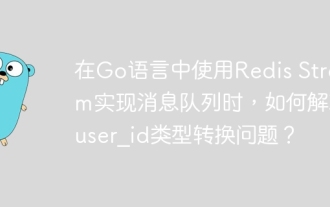
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
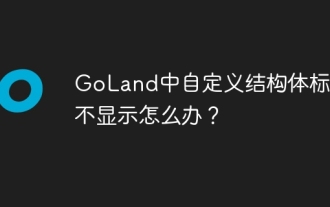
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
