


When Returning a Local Variable's Reference, Does It Return the Value or the Address (And Why Is This Dangerous)?
Local Variable Referencing Enigma: Returning Value or Address?
Many programmers may assume that returning references to local or temporary variables in functions is erroneous, resulting in undefined behavior. However, in certain situations, it may surprisingly succeed and modify the variable's value. Let's investigate this phenomenon with an illustrative code example:
#include <iostream> int& foo() { int i = 6; std::cout << &i << std::endl; // Prints the address of i before return return i; } int main() { int i = foo(); std::cout << i << std::endl; // Prints the value std::cout << &i << std::endl; // Prints the address of i after return }
If we execute this code, we observe curious behavior:
- The address of the local variable i in foo() is printed before returning, indicating its existence within the stack frame.
- Despite i being a local variable in foo(), its value is successfully modified when we assign the return value to a variable in main().
- The address of i in main() remains the same before and after returning, suggesting that the variable still resides in stack memory.
Explaining the Occurrence
This puzzling behavior can be attributed to a quirk in the way stack frames are handled in many implementations. When returning from a function, the stack frame is initially not immediately wiped.
In this example, the return type is int&, which means the function returns a reference to the address of i. The assignment int i = foo(); in main() stores the address of i in foo() in the variable i in main().
Simultaneously, the return instruction in foo() doesn't immediately release the stack frame. As a result, the variable i still exists in the stack memory, although it should have been destroyed. This allows i to be modified through its reference after returning.
Cautions and Implications
While this behavior may seem convenient, it is extremely dangerous and unreliable. Relying on the delayed stack frame destruction is a recipe for undefined behavior.
In well-defined code, you should ensure that local variables remain within the scope of their defining function and are promptly destroyed upon its termination. Failing to do so can lead to unpredictable consequences and errors.
The above is the detailed content of When Returning a Local Variable's Reference, Does It Return the Value or the Address (And Why Is This Dangerous)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


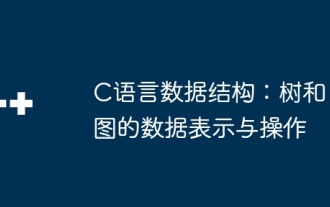
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
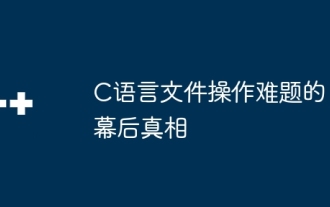
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
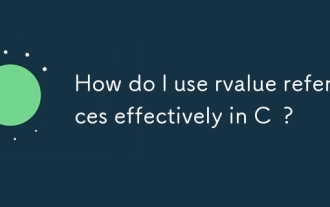
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
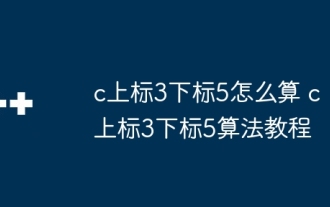
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
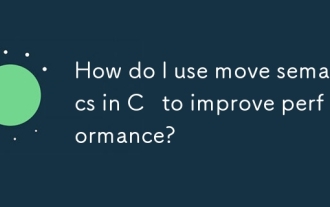
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
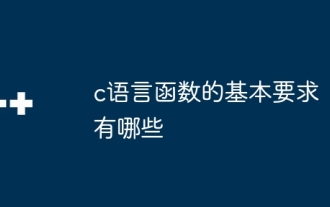
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
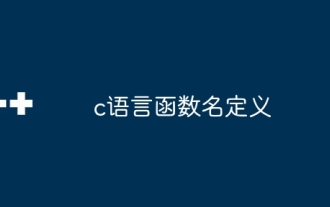
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
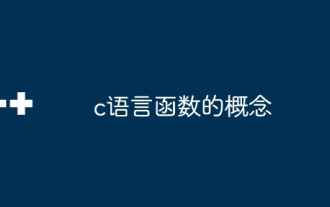
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
