Algorithmic Concepts in MongoDB Design
1. Sliding Window Concept
Application in MongoDB
// Sliding Window for Time-Series Data db.userActivity.aggregate([ // Sliding window for last 30 days of user engagement { $match: { timestamp: { $gte: new Date(Date.now() - 30 * 24 * 60 * 60 * 1000) } } }, { $group: { _id: { // Group by day day: { $dateToString: { format: "%Y-%m-%d", date: "$timestamp" }} }, dailyActiveUsers: { $addToSet: "$userId" }, totalEvents: { $sum: 1 } } }, // Sliding window aggregation to track trends { $setWindowFields: { sortBy: { "_id.day": 1 }, output: { movingAverageUsers: { $avg: "$dailyActiveUsers.length", window: { range: [-7, 0], unit: "day" } } } } } ])
Key Benefits
- Track rolling metrics
- Analyze time-based trends
- Efficient memory usage
2. Two-Pointer Technique
Schema Design Example
// Optimized Social Graph Schema { _id: ObjectId("user1"), followers: [ { userId: ObjectId("user2"), followedAt: ISODate(), interaction: { // Two-pointer like tracking mutualFollows: Boolean, lastInteractionScore: Number } } ], following: [ { userId: ObjectId("user3"), followedAt: ISODate() } ] } // Efficient Friend Recommendation function findPotentialConnections(userId) { return db.users.aggregate([ { $match: { _id: userId } }, // Expand followers and following { $project: { potentialConnections: { $setIntersection: [ "$followers.userId", "$following.userId" ] } } } ]); }
Optimization Techniques
- Reduce computational complexity
- Efficient relationship tracking
- Minimize full collection scans
3. Dynamic Programming (DP) Approach
Caching and Memoization
// DP-Inspired Caching Strategy { _id: "user_analytics_cache", userId: ObjectId("user1"), // Memoized computation results cachedMetrics: { last30DaysEngagement: { computedAt: ISODate(), totalViews: 1000, avgSessionDuration: 5.5 }, yearlyTrends: { // Cached computation results computedAt: ISODate(), metrics: { /* pre-computed data */ } } }, // Invalidation timestamp lastUpdated: ISODate() } // DP-like Incremental Computation function updateUserAnalytics(userId) { // Check if cached result is valid const cachedResult = db.analyticsCache.findOne({ userId }); if (shouldRecompute(cachedResult)) { const newMetrics = computeComplexMetrics(userId); // Atomic update with incremental computation db.analyticsCache.updateOne( { userId }, { $set: { cachedMetrics: newMetrics, lastUpdated: new Date() } }, { upsert: true } ); } }
4. Greedy Approach in Indexing
Indexing Strategy
// Greedy Index Selection db.products.createIndex( { category: 1, price: -1, soldCount: -1 }, { // Greedy optimization partialFilterExpression: { inStock: true, price: { $gt: 100 } } } ) // Query Optimization Example function greedyQueryOptimization(filters) { // Dynamically select best index const indexes = db.products.getIndexes(); const bestIndex = indexes.reduce((best, current) => { // Greedy selection of most selective index const selectivityScore = computeIndexSelectivity(current, filters); return selectivityScore > best.selectivityScore ? { index: current, selectivityScore } : best; }, { selectivityScore: -1 }); return bestIndex.index; }
5. Heap/Priority Queue Concepts
Distributed Ranking System
// Priority Queue-like Document Structure { _id: "global_leaderboard", topUsers: [ // Maintained like a min-heap { userId: ObjectId("user1"), score: 1000, lastUpdated: ISODate() }, // Continuously maintained top K users ], updateStrategy: { maxSize: 100, evictionPolicy: "lowest_score" } } // Efficient Leaderboard Management function updateLeaderboard(userId, newScore) { db.leaderboards.findOneAndUpdate( { _id: "global_leaderboard" }, { $push: { topUsers: { $each: [{ userId, score: newScore }], $sort: { score: -1 }, $slice: 100 // Maintain top 100 } } } ); }
6. Graph Algorithms Inspiration
Social Network Schema
// Graph-like User Connections { _id: ObjectId("user1"), connections: [ { userId: ObjectId("user2"), type: "friend", strength: 0.85, // Inspired by PageRank-like scoring connectionScore: { mutualFriends: 10, interactions: 25 } } ] } // Connection Recommendation function recommendConnections(userId) { return db.users.aggregate([ { $match: { _id: userId } }, // Graph traversal-like recommendation { $graphLookup: { from: "users", startWith: "$connections.userId", connectFromField: "connections.userId", connectToField: "_id", as: "potentialConnections", maxDepth: 2, restrictSearchWithMatch: { // Avoid already connected users _id: { $nin: existingConnections } } } } ]); }
Scalability Considerations
Key Principles
-
Algorithmic Efficiency
- Minimize collection scans
- Use indexing strategically
- Implement efficient aggregation
-
Distributed Computing
- Leverage sharding
- Implement smart partitioning
- Use aggregation pipeline for distributed computing
-
Caching and Memoization
- Cache complex computations
- Use time-based invalidation
- Implement incremental updates
Key Skills
- Understand data access patterns
- Know indexing strategies
- Recognize query complexity
- Think about horizontal scaling
The above is the detailed content of Algorithmic Concepts in MongoDB Design. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


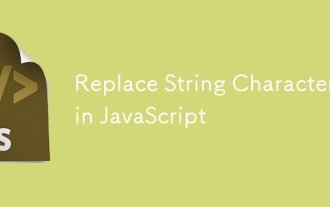
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
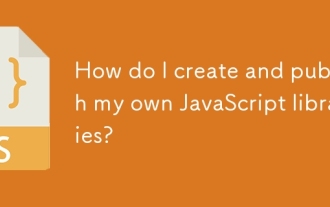
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
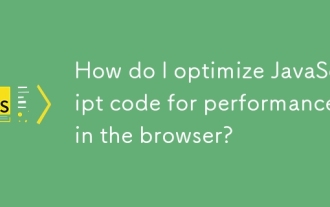
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
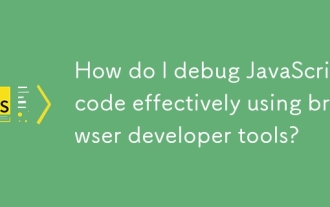
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
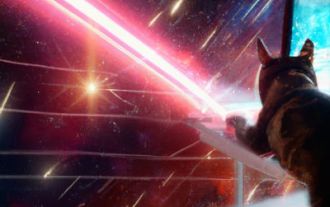
This article outlines ten simple steps to significantly boost your script's performance. These techniques are straightforward and applicable to all skill levels. Stay Updated: Utilize a package manager like NPM with a bundler such as Vite to ensure
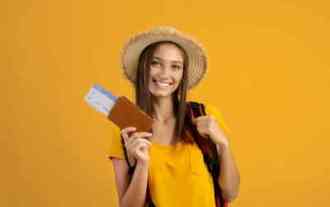
Sequelize is a promise-based Node.js ORM. It can be used with PostgreSQL, MySQL, MariaDB, SQLite, and MSSQL. In this tutorial, we will be implementing authentication for users of a web app. And we will use Passport, the popular authentication middlew
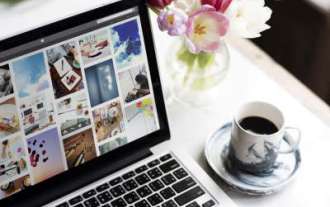
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
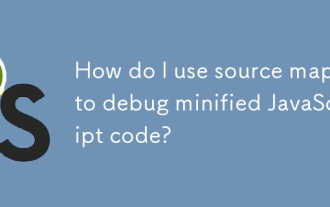
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
