


How Can I Save Files Directly from My Browser Using HTML5 and JavaScript?
Saving Files in HTML5/JavaScript: A Complete Guide
Introduction
Web developers have long sought ways to generate and save files using HTML5 and JavaScript. This capability allows users to download and share data without involving server-side processes. This article will provide a comprehensive guide on how to achieve this using the built-in features of HTML5.
Problem Statement
Many developers encounter the need to convert files into more efficient formats. However, using servers for this process introduces unnecessary overhead. Hence, the question arises: is there a way to facilitate file downloads using pure JavaScript?
Solution: The HTML5 Save File Method
The issue can be resolved using the following steps:
- Create a Blob Object: A Blob object represents a file-like data structure and can be used to save data in desired formats.
- Create a URL Object: A URL object represents a file path and can be used to generate a downloadable file from the Blob object.
- Create a Download Anchor: Create an anchor tag with the URL pointing to the file-like data structure.
- Trigger the Download: Simulate a click event on the anchor tag to initiate the download.
Example Code:
The following code demonstrates this technique:
function download(filename, data) { // Create a Blob object const blob = new Blob([data], { type: 'text/plain' }); // Create a URL object const url = URL.createObjectURL(blob); // Create a download anchor const anchor = document.createElement('a'); anchor.setAttribute('href', url); anchor.setAttribute('download', filename); // Trigger the download anchor.click(); // Remove the anchor from the DOM (optional) document.body.removeChild(anchor); }
Usage:
To use this method, simply call the download() function with the desired filename and data.
Conclusion
This article has provided a thorough explanation of how to generate and save files using HTML5 and JavaScript. The presented methods allow developers to create file-downloading capabilities within their web applications without relying on server-side processes.
The above is the detailed content of How Can I Save Files Directly from My Browser Using HTML5 and JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










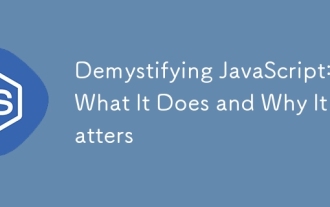
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
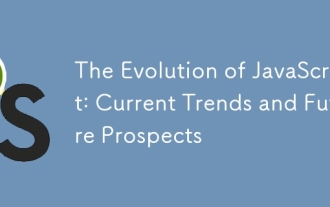
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
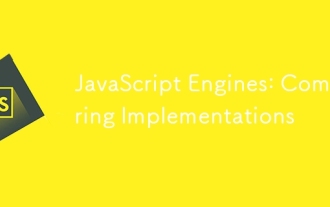
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
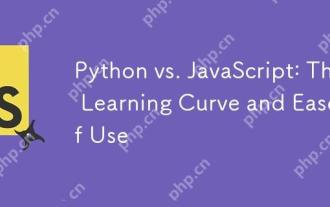
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
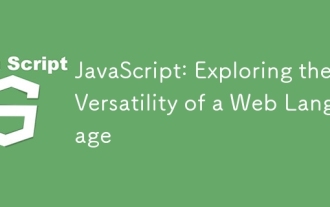
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
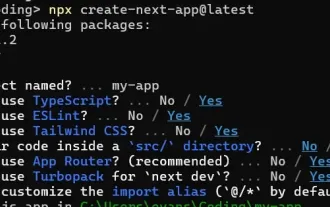
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
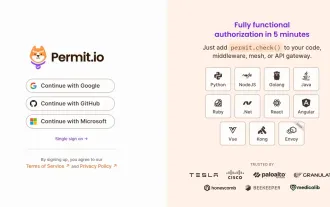
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
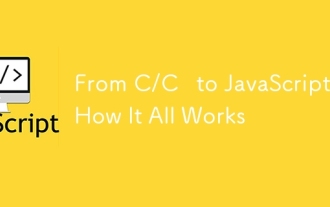
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
