How Can I Perform HTTP GET Requests in JavaScript?
Performing HTTP GET Requests in JavaScript
In JavaScript, there are several methods to perform HTTP GET requests. One common approach is through theXMLHttpRequest (XHR) object, which establishes an asynchronous connection with the server. This connection allows you to send and receive data without blocking the main thread.
To use XHR for a GET request, you can follow these steps:
-
Create an XHR object:
1
var
xhr =
new
XMLHttpRequest();
Copy after login -
Open the connection:
1
xhr.open(
"GET"
, theUrl);
Copy after login -
Send the request:
1
xhr.send();
Copy after login -
When the response is ready, handle the response:
1
2
3
4
5
6
7
xhr.onload =
function
() {
if
(xhr.status == 200) {
// Success! Handle the response here
}
else
{
// Error handling logic
}
};
Copy after loginIn modern JavaScript, the Fetch API is another popular option for performing HTTP requests. It offers a more straightforward syntax and returns a Promise for handling the response:
1 2 3 4 5 6 7 |
|
For Mac OS X Dashcode widgets, the XHR method is recommended as it is fully supported within the framework. By leveraging XHR or Fetch API, you can seamlessly perform HTTP GET requests in your JavaScript code.
The above is the detailed content of How Can I Perform HTTP GET Requests in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
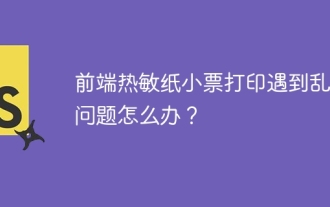
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
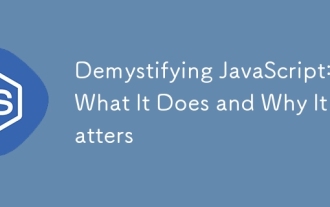
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
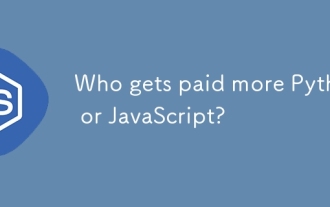
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
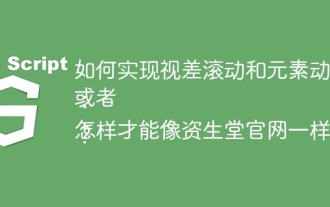
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
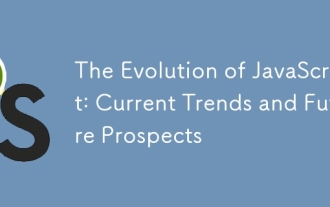
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
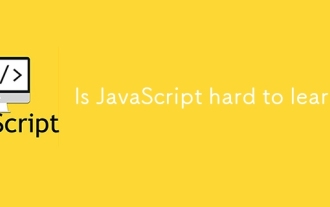
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
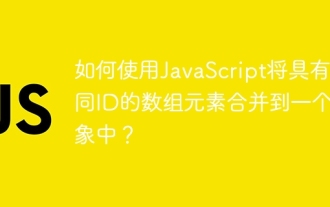
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
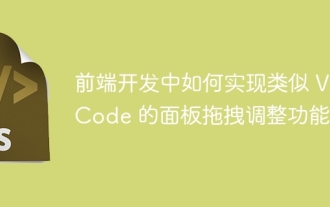
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
