


How Can Swing KeyBindings Enable Two Players to Control Separate Paddles Using Different Keys in a Java Game?
Using Key Bindings
To address the main issue of allowing two players to control separate paddles using different keys, the suggested solution is to use Swing KeyBindings. This approach provides the following advantages:
- Key bindings are separate from the rest of the game logic and allow for greater flexibility.
- Key bindings are responsive even when the game is not in focus, unlike key listeners.
Code Example
The provided code example demonstrates how to implement key bindings in your Java application:
//Create a GameKeyBindings object associated with the game panel and the two paddle entities. GameKeyBindings gameKeyBindings = new GameKeyBindings(gp, player1Entity, player2Entity);
Within the GameKeyBindings class, key bindings are defined for each paddle's movement:
//Key binding for Player 1's paddle: Up arrow key gp.getInputMap(JComponent.WHEN_IN_FOCUSED_WINDOW).put(KeyStroke.getKeyStroke(KeyEvent.VK_W, 0, false), "W pressed"); gp.getActionMap().put("W pressed", new AbstractAction() { @Override public void actionPerformed(ActionEvent ae) { entity.UP = true; } }); //Key binding for Player 2's paddle: Down arrow key gp.getInputMap(JComponent.WHEN_IN_FOCUSED_WINDOW).put(KeyStroke.getKeyStroke(KeyEvent.VK_DOWN, 0, false), "down pressed"); gp.getActionMap().put("down pressed", new AbstractAction() { @Override public void actionPerformed(ActionEvent ae) { entity2.DOWN = true; } }); // Similar key binding definitions for releasing the keys.
Explanation of Collections.synchronizedSet(new HashSet
The Collections.synchronizedSet(new HashSet
In the provided code, the synchronized set is used to track which keys are currently pressed. This information is required for the game logic to determine which paddles should be moving.
Additional Information
- It is not necessary to toggle separate threads to achieve the desired functionality.
- Key bindings are a more robust and elegant solution for handling key input than using key listeners.
- The provided code example is a simple and straightforward implementation; further optimizations and enhancements can be added as needed.
The above is the detailed content of How Can Swing KeyBindings Enable Two Players to Control Separate Paddles Using Different Keys in a Java Game?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










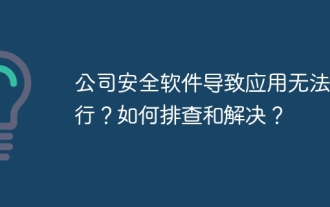
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
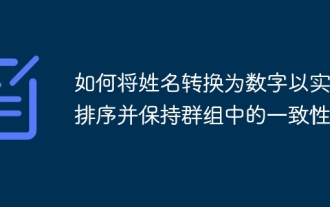
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
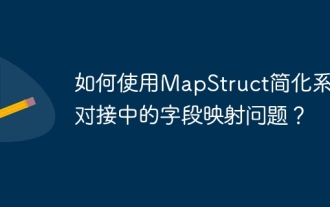
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
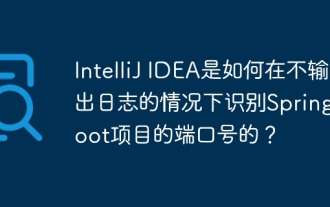
Start Spring using IntelliJIDEAUltimate version...
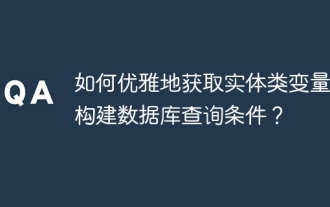
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
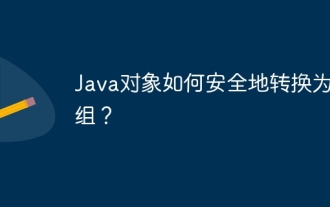
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
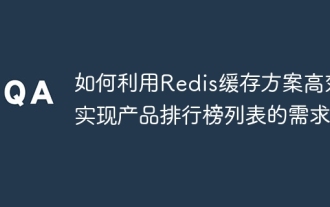
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
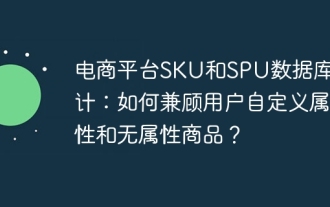
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
