Can C Constructors Be Chained, and If So, How?
Dec 21, 2024 am 02:58 AMCan Constructor Chaining Be Done in C ?
In C#, the ability to call constructors in a specific order is a common practice. As a C# developer seeking to replicate this functionality in C , the question arises: can it be achieved through constructor chaining?
C 11 and Onwards
Rejoice! C 11 introduces a feature known as delegating constructors that mimics the constructor chaining seen in C#. Here's how it's written:
class Foo { public: Foo(char x, int y) {} Foo(int y) : Foo('a', y) {} };
C 03: A Different Approach
Despite the absence of direct constructor chaining in C 03, two workarounds exist:
Default Parameters: Combine constructors using default parameters.
class Foo { public: Foo(char x, int y=0); // Combines two constructors (char) and (char, int) };
Init Method: Utilize a shared initialization method.
class Foo { public: Foo(char x); Foo(char x, int y); private: void init(char x, int y); }; Foo::Foo(char x) : init(x, int(x) + 7) {} Foo::Foo(char x, int y) : init(x, y) {} void Foo::init(char x, int y) {}
In conclusion, C 11 enables true constructor chaining, while C 03 offers workarounds like default parameters and init methods to achieve similar functionality.
The above is the detailed content of Can C Constructors Be Chained, and If So, How?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
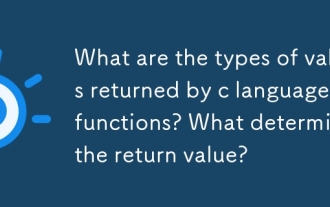
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
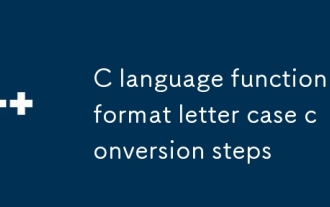
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
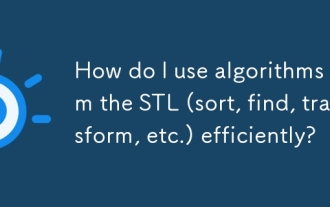
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
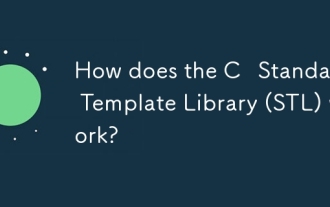
How does the C Standard Template Library (STL) work?
