


How Can I Avoid Unexpected Behavior When Using Go's `interface{}` with `json.Unmarshal`?
Golang interface{} Type Misunderstanding
When using the interface{} type as a function parameter, developers often encounter unexpected behavior, especially when interacting with JSON data through json.Unmarshal.
The Issue:
In Go, interface{} is a type that can hold any value and type. However, it's commonly misunderstood as a typeless container. This misunderstanding arises in situations like:
func test(i interface{}) { j := []byte(`{ "foo": "bar" }`) json.Unmarshal(j, &i) fmt.Println(i) } func main() { test(Test{}) }
Expected Behavior:
This code attempts to unmarshal JSON data into a Test struct. However, it results in an unexpected conversion of the struct to a map[string]interface{} type.
Explanation:
json.Unmarshal cannot unmarshal JSON into a non-pointer value. Since i is an interface{} holding a Test struct, it's passed as an address (&i) to allow modification. However, json.Unmarshal views the interface{} pointer as a pointer to an interface{} value containing the Test struct. Unable to unmarshal into a non-pointer, it defaults to creating a new map[string]interface{} value for the unmarshaled JSON.
The Right Approach:
To resolve this misunderstanding, consider the following scenario:
func test(i *interface{}) { j := []byte(`{ "foo": "bar" }`) json.Unmarshal(j, i) fmt.Println(*i) } func main() { var i *Test test(&i) }
Explanation:
- Pass a pointer to the interface{} parameter, effectively wrapping a pointer in an interface{}.
- Inside the function, dereference the interface{} pointer and pass it directly to json.Unmarshal.
- Since i holds a pointer to the Test struct, json.Unmarshal correctly unmarshals the JSON data into the struct.
Conclusion:
interface{} is a type that can hold any value, but it's not simply a placeholder for any type. To avoid unexpected behavior, properly handle pointers when using interface{} as function parameters, especially with JSON operations.
The above is the detailed content of How Can I Avoid Unexpected Behavior When Using Go's `interface{}` with `json.Unmarshal`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
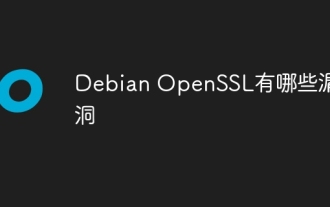
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
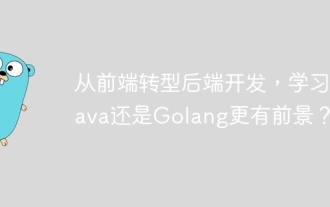
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
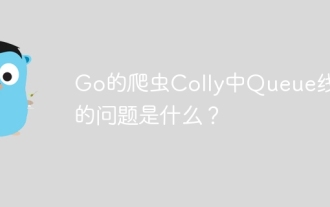
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
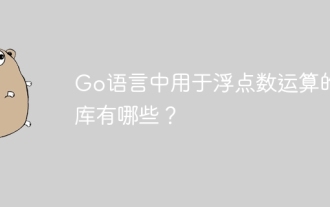
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
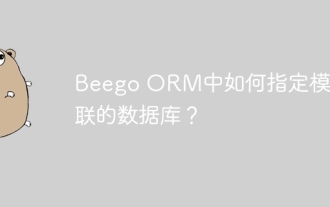
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
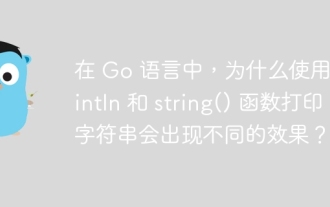
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
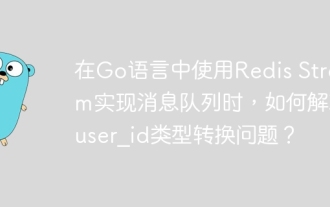
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
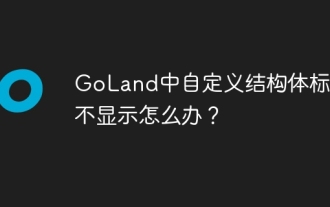
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
