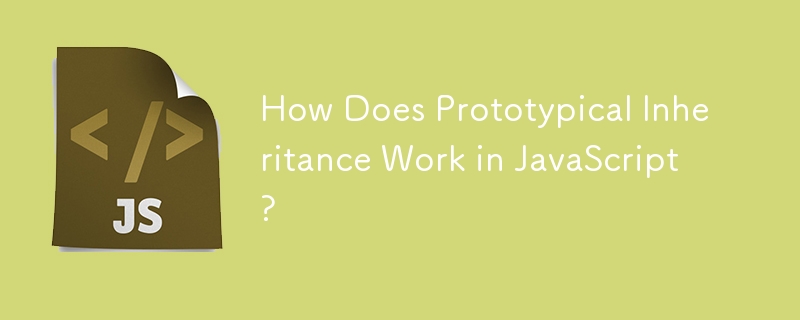
Prototypical Inheritance in JavaScript
Prototypical inheritance is a crucial concept in JavaScript's object-oriented programming that allows objects to inherit properties and methods from other objects.
Understanding the Chain
JavaScript follows a prototypical inheritance model, wherein an object inherits from its prototype object. If a property or method does not exist on the object itself, JavaScript searches the prototype object for it. This chain continues until the end of the prototype chain, typically reaching the built-in Object object.
Adding Properties and Methods
-
.__proto__: Used to set the prototype object directly.
-
Object.create(prototype): Creates a new object with the specified prototype.
Instance vs. Prototype Properties
-
Instance properties: Unique to each object instance. Accessed via the instance itself (e.g., object.property).
-
Prototype properties: Shared among all objects that inherit from the same prototype. Accessed via the prototype object (e.g., Object.prototype.property).
Shadowing and Overriding
-
Shadowing: Assigning a property directly to an instance overrides the prototype property.
-
Overriding: Altering the prototype property itself changes the behavior for all objects that inherit from that prototype.
Creating Constructors
-
Constructor functions: Set the prototype of new objects, providing a way to initialize instance properties.
-
this: Represents the object being created.
Inheritance Patterns
-
Classical inheritance: Uses constructors and this to set prototypes (e.g., function Hamster() { this.food = []; }).
-
Object.create inheritance: Explicitly sets the prototype using Object.create (e.g., var mini = Object.create(Hamster.prototype)).
-
Mixins: Provide additional functionality to objects without creating a new prototype (e.g., Mixin.mix(Cat, Movable)).
Concerns
-
Accidental overrides: Modifying prototype properties unintentionally can affect multiple objects.
-
Prototype pollution: Adding properties to the built-in Object prototype can interfere with other JavaScript code.
Private Variables
JavaScript does not have true private variables, but conventions and techniques can emulate privacy.
-
Naming conventions: Prefix private properties with underscores (e.g., _privateProperty).
-
Closures: Encapsulate private properties within functions to limit their scope.
Recap
Prototypical inheritance forms the basis of object-oriented programming in JavaScript. It enables objects to share and reuse properties and methods, offering flexibility and code reusability. However, it's essential to understand the inheritance mechanisms and potential pitfalls to avoid issues and maintain code quality.
The above is the detailed content of How Does Prototypical Inheritance Work in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!