


How Can I Reliably Detect the Existence of Specific Member Variables in a C Class?
Dec 21, 2024 am 10:13 AMDetecting the Existence of Specific Member Variables in a Class
In code, checking for the presence of particular member variables in a class can be challenging. This can be crucial when implementing generic algorithms or working with classes with varying member names.
Problem Statement:
The provided code aims to address this issue by checking for the existence of "x" (or "X") and "y" (or "Y") in a class template argument. This is particularly useful in classes like MFC's CPoint or GDI 's PointF, where member variable names differ.
The original solution used different templated functions for "x" and "X." However, this resulted in compilation issues in Visual Studio.
Universal Solution:
To achieve a universal solution, we can utilize SFINAE (Substitution Failure is Not an Error). The modified code below demonstrates this approach:
template <typename T> struct HasX : std::false_type { }; template <typename T> struct HasX<T, decltype((void)T::x, 0)> : std::true_type { };
This code exploits SFINAE by attempting to substitute "T::x" in the "decltype(...)." If "T::x" is valid, the specialization for HasX<T, decltype((void) T::x, 0)> will be used, resulting in HasX<T>::value being true; otherwise, it will be false.
This solution is not restricted to C 11 and provides a generic method for detecting member variables in any class.
The above is the detailed content of How Can I Reliably Detect the Existence of Specific Member Variables in a C Class?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
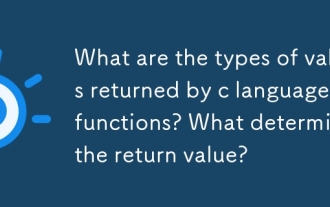
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
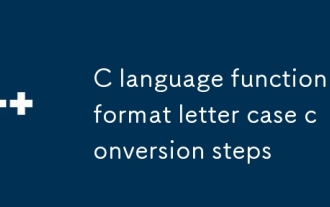
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
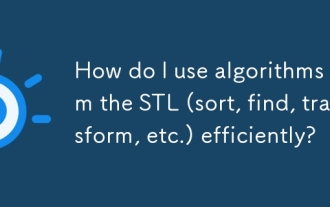
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
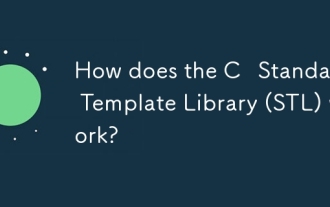
How does the C Standard Template Library (STL) work?
