How Can I Manually Raise Exceptions in Python?
How do I manually raise (throw) an exception in Python?
Problem Statement
How can I raise an exception in Python that can be caught later using an except block?
Solution
Steps to Raise an Exception:
- Use the Specific Exception Class: Identify the most appropriate exception class that semantically represents the error.
- Create an Exception Instance: Instantiate the Exception class with a meaningful message using the constructor syntax: raise ExceptionClass(message).
- Pass Additional Arguments: You can optionally pass additional arguments to the exception constructor, accessible via the args attribute.
Best Practices
Raising Exceptions
- Raise Specific Exceptions: Avoid raising generic Exception objects. Choose the most specific subclass that accurately describes the issue.
- Provide Meaningful Messages: Include a clear and concise error message in your exception instances.
- Preserve Stack Traces: Use raise ValueError('Error message') to preserve stack traces in captured exceptions.
Catching Exceptions
- Use Specific Catches: Handle errors by catching specific exceptions that may get raised. Avoid catching generic Exception if possible.
- Re-raise Exceptions: If you encounter an error within an except clause that you want to handle at a higher level, use raise with no arguments to re-raise it while preserving the stack trace.
Exception Chaining
- Python 3 Enhancement: In Python 3, you can use raise RuntimeError('specific message') from error to chain exceptions, preserving tracebacks and optionally changing the exception type.
Cautions
- Avoid Modifying Tracebacks: Manipulating exception tracebacks using sys.exc_info() is discouraged due to potential errors and Python version compatibility issues.
- Deprecation Warning: Avoid using raise ValueError, 'message' (Python 2) and raise 'message' (Python 2.4 and lower) as they can raise unintended exceptions.
Example Usage
def raise_custom_exception(): raise CustomException("This custom exception was intentionally raised.")
Custom Exception Classes
In situations where no built-in exception class fits the specific error scenario, you can create custom exception classes by subclassing the appropriate base exception.
class MyAppError(Exception): def __init__(self, message): super().__init__(message)
The above is the detailed content of How Can I Manually Raise Exceptions in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
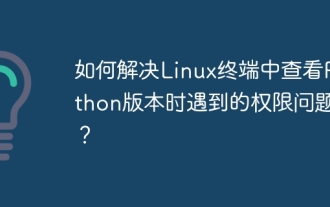
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
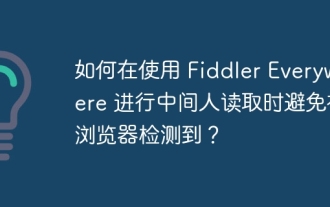
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
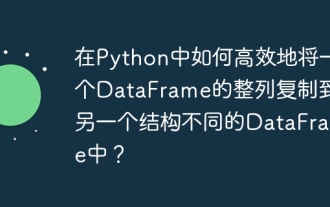
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
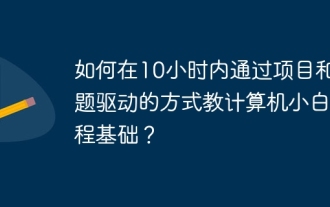
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
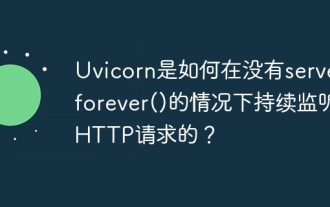
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
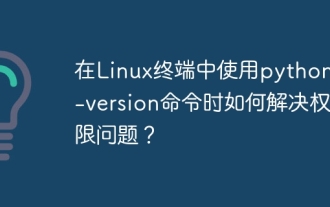
Using python in Linux terminal...
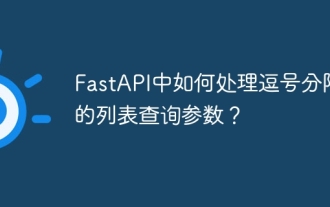
Fastapi ...
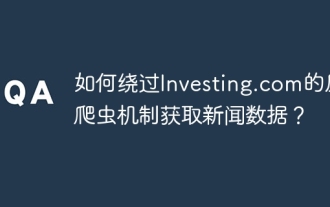
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
