


What are the Limitations and Unexpected Behaviors of Java's Arrays.asList() Method with Primitive Arrays?
Arrays.asList() Behavior and Limitations
In Java, the Arrays.asList() method creates an unmodifiable list from an array. It supports varargs arguments, which are convenient for passing an array of values. However, using Arrays.asList() with primitive array types can lead to unexpected results.
Consider the following code:
1 2 3 4 5 |
|
In the first example, Arrays.asList() successfully creates a List
Why the Unexpected Behavior?
Java generics do not support primitive types. Instead, they use wrapper classes like Integer and Float to represent these values. When Arrays.asList() is invoked with a primitive array, it attempts to create a list of the corresponding wrapper class. However, since there is no List
How to Correct It
To obtain a List
1 2 3 4 5 6 |
|
Why Autoboxing Doesn't Work for Arrays
Autoboxing applies only to individual primitives, not entire arrays. For instance, the expression Integer.valueOf(intArray) will not autobox all elements of intArray into Integer objects. Instead, it will result in a compile-time error.
Alternative Solutions
Alternatively, you can use other libraries that provide methods for converting primitive arrays to lists of wrapper classes. For example, the Guava library offers the Ints.asList() method, which wraps a primitive int[] into a List
The above is the detailed content of What are the Limitations and Unexpected Behaviors of Java's Arrays.asList() Method with Primitive Arrays?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
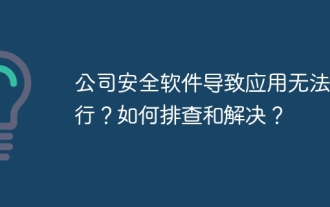
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
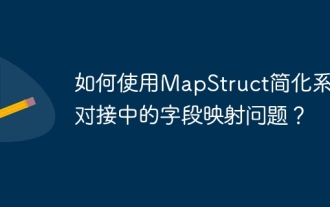
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
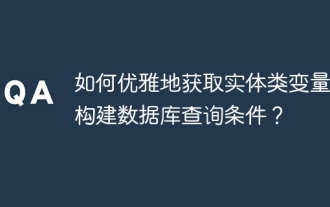
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
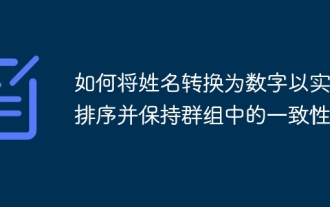
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
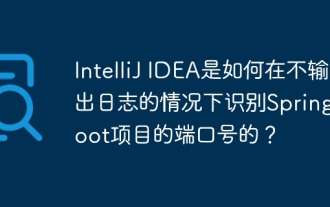
Start Spring using IntelliJIDEAUltimate version...
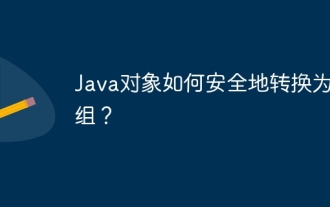
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
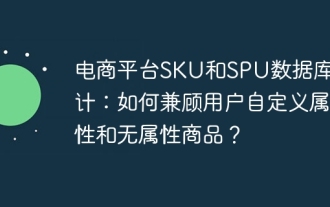
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
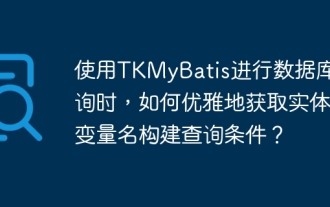
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
