


How to Set HTTP Response Headers in a Go Web Server using gorilla/mux?
Setting Headers in a Go HTTP Server
When developing web APIs, it's often necessary to set HTTP headers on response objects to control the behavior of the browser. In Go, using gorilla/mux and net/http to build a web server, setting response headers is a straightforward process.
Consider this example, which aims to allow cross-domain AJAX requests by setting the "Access-Control-Allow-Origin" header to "*":
package main import ( "net/http" "github.com/gorilla/mux" ) func saveHandler(w http.ResponseWriter, r *http.Request) { // allow cross domain AJAX requests w.Header().Set("Access-Control-Allow-Origin", "*") } func main() { r := mux.NewRouter() r.HandleFunc("/save", saveHandler) http.Handle("/", r) http.ListenAndServe(":"+port, nil) }
The key to setting response headers is the Header() method on the ResponseWriter object:
- w.Header().Set(): Sets the header to the specified value.
In this case, the code uses w.Header().Set("Access-Control-Allow-Origin", "*") to add the desired header and value. The result will be an HTTP response with the "Access-Control-Allow-Origin" header set to "*".
This simple method allows you to control the HTTP headers sent with your server's responses, enabling features such as Cross-Origin Resource Sharing (CORS).
The above is the detailed content of How to Set HTTP Response Headers in a Go Web Server using gorilla/mux?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




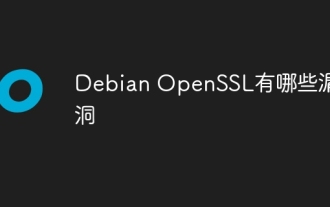
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
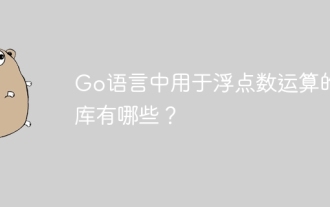
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
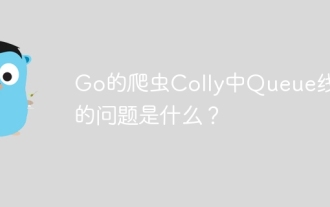
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
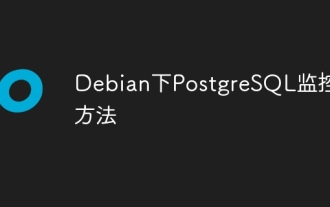
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
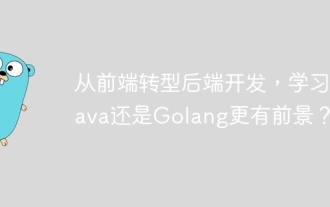
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
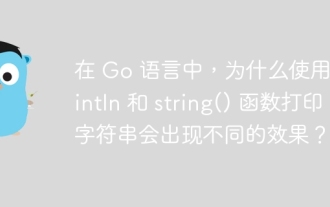
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
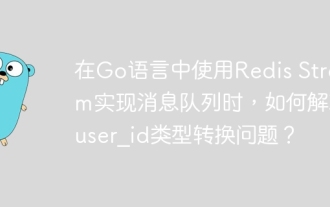
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
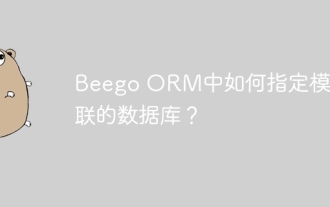
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
