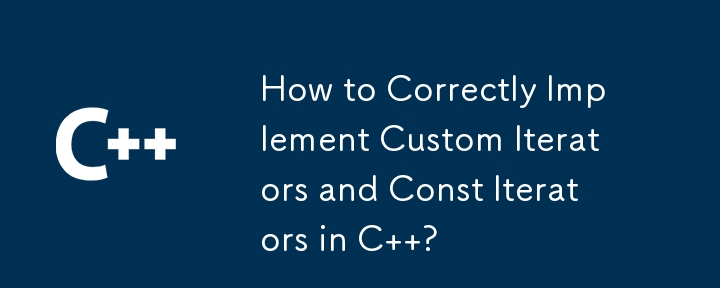
Correct Implementation of Custom Iterators and Const Iterators
Creating custom iterators and const iterators for a custom container class can be a daunting task. Here are some guidelines and best practices to ensure their correctness.
Iterator Guidelines:
-
Select the Appropriate Iterator Type: Consider the operations you plan to perform on the container elements and determine the suitable iterator type (e.g., input, output, forward).
-
Utilize Base Iterator Classes: Leverage base iterator classes like std::iterator and its derivatives. They handle essential type definitions and standard functionality.
-
Template-Based Design: Use a template-based iterator class parameterized by value type, pointer type, and reference type. This approach avoids code duplication.
Code Duplication Mitigation:
To minimize code overlap between const iterators and iterators:
-
Parametrize: Define a template class for the iterator that accepts both const and non-const pointer types.
-
Create Type Aliases: Establish distinct type aliases for const and non-const iterators.
Additional Considerations:
- Refer to the standard library reference for further details on iterator implementations.
- Since C 17, std::iterator is deprecated. See the discussion here for further guidance.
The above is the detailed content of How to Correctly Implement Custom Iterators and Const Iterators in C ?. For more information, please follow other related articles on the PHP Chinese website!