How Can I Return a `std::unique_ptr` Without Using `std::move`?
Returning std::unique_ptr without std::move: How Is It Possible?
Despite the prohibition of copy construction in std::unique_ptr
The Exception: Copy Elision
C allows copy elision under specific circumstances, as defined in 12.8 §34 and §35. One such case is when a non-volatile automatic object with the same cv-unqualified type as the function's return type is returned. In this scenario, the compiler is permitted to elide the copy construction process. This elision is applied to both copies and moves.
The Implementation of Copy Elision
When copy elision is employed in a return statement, the compiler first considers the object as an rvalue, even if it is an lvalue, for the purpose of overload resolution. Consequently, if a move constructor is available, it is selected, but no actual move operation is performed. This results in an empty move constructor call that acts as a placeholder and maintains the ownership semantics of the unique pointer.
Example
The following code demonstrates the phenomenon:
unique_ptr<int> foo() { unique_ptr<int> p( new int(10) ); return p; // Line 1, copy elision applied } int main() { unique_ptr<int> p = foo(); cout << *p << endl; return 0; }
In line 1, the returned unique_ptr
Conclusion
This exception to the copy constructor prohibition is specifically allowed by the C language specification to facilitate efficient and concise return statements. However, it is important to note that copy elision is an optimization that the compiler may or may not perform. As such, it is generally recommended to explicitly use std::move in return statements to ensure the intended behavior.
The above is the detailed content of How Can I Return a `std::unique_ptr` Without Using `std::move`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


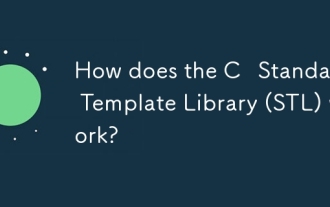
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
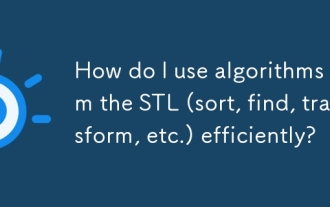
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
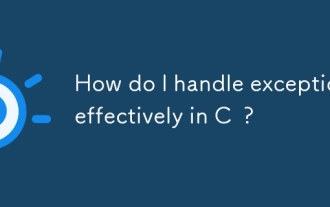
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
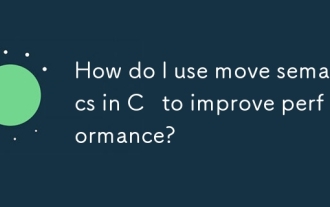
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
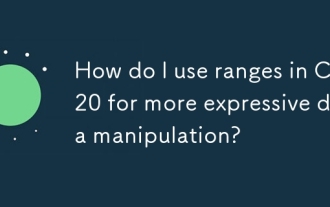
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
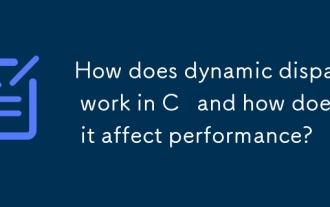
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
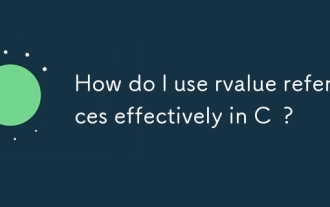
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
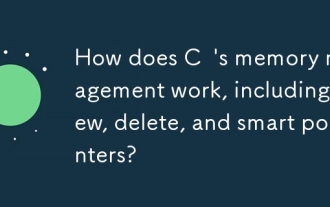
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
