


Why Do I Get Cryptic Errors When Using User-Defined Types as Keys in `std::map`, and How Can I Fix Them?
How to Use std::Maps with User-Defined Types as Keys: Understanding the Cryptic Error and Alternative Approaches
Introduction
When attempting to utilize STL maps with user-defined classes as keys, programmers may encounter cryptic error messages, hindering their progress. This article delves into the reason behind this error and explores alternative approaches to employ std::maps effectively.
The Cryptic Error and Reason
As illustrated in the example provided, using user-defined types as map keys raises an error stemming from the compiler's inability to locate a suitable comparison operator for the specific type. In this instance, std::map relies on the availability of the operator< function to determine the ordering of elements.
Alternative Approaches
1. Comparator Function Object:
One method to circumvent the error is to define a comparator function object, which implements the operator< functionality. This approach allows you to establish comparison logic without exposing it via an operator overload on the user-defined class.
For example, the following code snippet employs a comparator function object to compare and order Class1 instances:
struct Class1Compare { bool operator() (const Class1& lhs, const Class1& rhs) const { return lhs.id < rhs.id; } }; std::mapc2int; 2. Specialization of std::less:
An alternative approach involves specializing the std::less template to provide the comparison semantics specifically for the user-defined type. This ensures seamless integration with std::map's default comparison behavior without exposing an explicit operator< overload:
namespace std { template<> struct less{ bool operator() (const Class1& lhs, const Class1& rhs) const { return lhs.id < rhs.id; } }; } By adopting either of these alternatives, programmers can effectively use std::maps with user-defined type keys, eliminating the need for explicit operator< overloads while maintaining the flexibility and efficiency offered by STL maps.
The above is the detailed content of Why Do I Get Cryptic Errors When Using User-Defined Types as Keys in `std::map`, and How Can I Fix Them?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


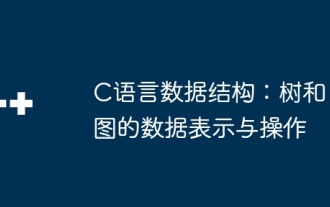
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
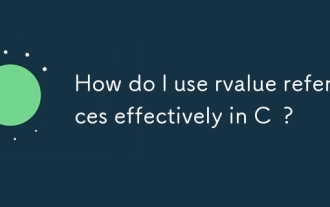
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
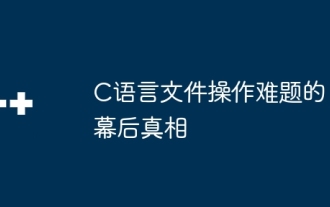
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
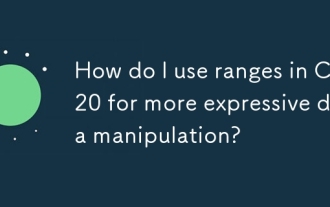
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
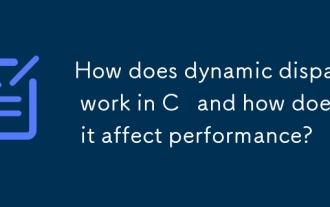
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
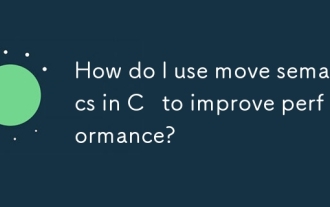
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
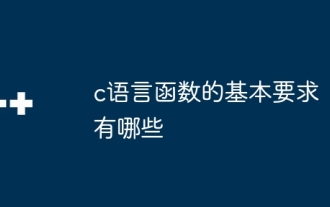
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
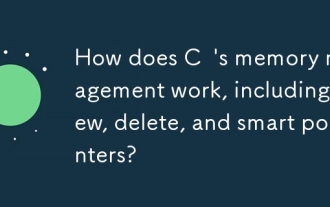
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
