


How to Remap Pandas Column Values Using a Dictionary While Keeping NaN Values?
Remapping Values in Pandas Column Using Dictionary While Preserving NaN
In the context of processing dataframes, it is often necessary to alter values in a specific column based on defined mappings. Consider a scenario where you have a dictionary containing predefined value translations, such as di = {1: "A", 2: "B"}, and you want to apply these mappings to a pandas column named col1. The goal is to modify the values in col1 accordingly, while leaving NaN values untouched.
One highly effective approach to achieve this transformation is by leveraging pandas' .replace method. This method allows for the replacement of specific values or ranges with designated target values. Here's how you can implement it:
import pandas as pd import numpy as np # Example DataFrame df = pd.DataFrame({'col2': {0: 'a', 1: 2, 2: np.nan}, 'col1': {0: 'w', 1: 1, 2: 2}}) # Mapping dictionary di = {1: "A", 2: "B"} # Apply value remapping using .replace df.replace({"col1": di}, inplace=True) # Output DataFrame with remapped values while preserving NaN print(df)
In this example, the .replace method takes a dictionary as an argument, where the keys represent the original values in col1, and the values represent the desired remapped values. By setting the inplace parameter to True, the original dataframe is modified directly, saving the need for reassignment.
Alternatively, if you prefer to apply the transformation specifically to the col1 Series, you can use the following syntax:
df["col1"].replace(di, inplace=True)
This approach ensures that NaN values remain unaffected, as NaN is not a key in the mapping dictionary.
The above is the detailed content of How to Remap Pandas Column Values Using a Dictionary While Keeping NaN Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










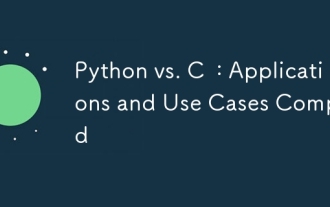
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
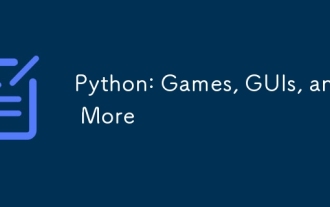
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
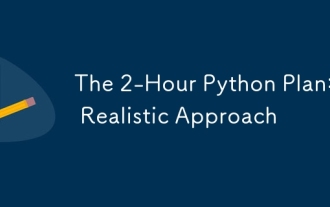
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
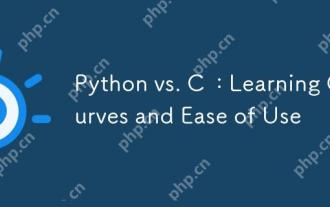
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
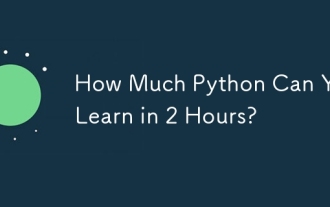
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
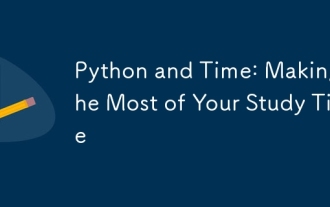
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
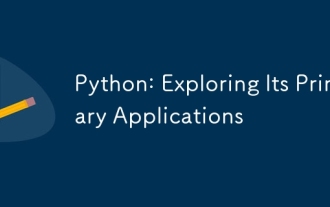
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
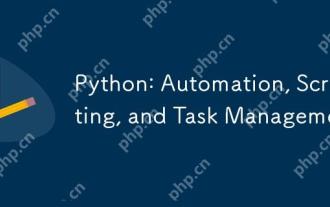
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
