How Does Python Implement Its Lists Internally?
Python List Implementation: Unraveling the Enigma
Python lists, an integral aspect of the language, store collections of elements of any type. Many developers have speculated about their underlying implementation, but definitive answers have remained elusive. This article delves into the depths of Python's C code to uncover the truth behind list realization.
Examining the header file listobject.h, we discover the fundamental structure of a Python list:
typedef struct { PyObject_HEAD Py_ssize_t ob_size; /* Vector of pointers to list elements. list[0] is ob_item[0], etc. */ PyObject **ob_item; /* ob_item contains space for 'allocated' elements. The number * currently in use is ob_size. * Invariants: * 0 ≤ ob_size ≤ allocated * len(list) == ob_size * ob_item == NULL implies ob_size == allocated == 0 */ Py_ssize_t allocated; } PyListObject;
This code reveals that Python lists are indeed implemented as vectors or arrays. Specifically, they utilize an overallocation strategy, which means that memory is allocated in advance for potential additions to the list.
If the list reaches its allocated limit, the resize code in listobject.c expands the array by allocating:
new_allocated = (newsize >> 3) + (newsize < 9 ? 3 : 6); new_allocated += newsize;
where newsize represents the requested size, whether for extending by an arbitrary number of elements or simply appending one.
Furthermore, the Python FAQ provides additional insights into the list implementation, highlighting its dynamic and efficient nature, capable of resizing as needed while preserving performance.
The above is the detailed content of How Does Python Implement Its Lists Internally?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
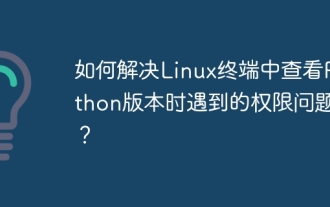
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
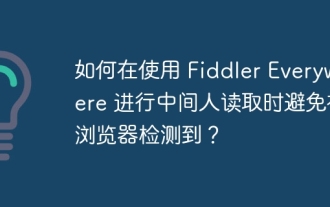
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
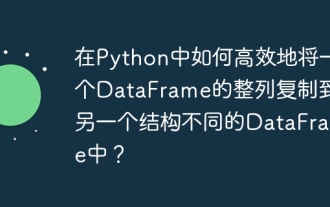
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
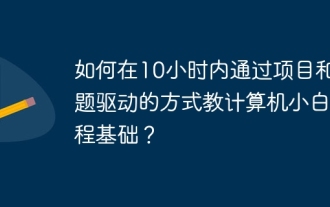
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
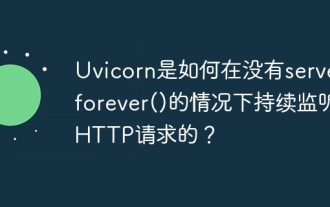
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
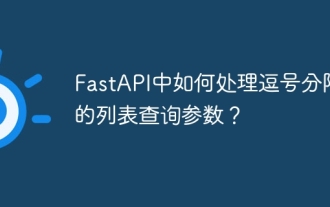
Fastapi ...
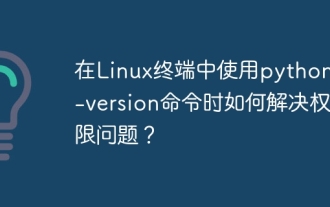
Using python in Linux terminal...
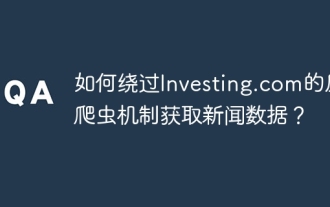
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
