


How to Effectively Remove Top-Level Containers in Swing to Prevent Memory Leaks?
Removing Top-Level Containers at Runtime
Problem
Creating and displaying multiple top-level containers (e.g., JDialogs, JFrames) can lead to memory leaks in Swing applications. Even after disposing of these containers, the Java Virtual Machine (JVM) may not reclaim the occupied memory until the respective WINDOW_CLOSING event is processed. This issue can lead to continuous memory consumption and potential OutOfMemoryErrors.
Solution
To address this issue, the following steps should be followed:
- Dispose the Top-Level Containers: Properly dispose of the top-level containers by invoking the dispose() method. This allows the host platform to reclaim memory associated with the heavyweight peer of the container.
- Handle the WINDOW_CLOSING Event: Ensure that the WINDOW_CLOSING event is processed on the EventQueue. This can be done by dispatching a WindowEvent of type WINDOW_CLOSING to the container.
- Invoke Garbage Collection: Invoke the gc() method to suggest the JVM to perform garbage collection.
Additional Considerations
Garbage Collection is a Suggestion: It's important to note that invoking gc() is merely a suggestion to the JVM. The JVM may choose to initiate garbage collection immediately or defer it for later based on various factors.
Allocate Memory Conservatively: When memory is constrained, consider allocating memory conservatively. This may involve using lightweight components instead of heavyweight ones, or adopting a headless approach with 2D graphics using lightweight components only.
Implementation
The following code demonstrates the solution:
// Create a top-level container (e.g., JDialog, JFrame) and dispose of it later. JDialog dialog = new JDialog(); dialog.dispose(); // Dispatch the WINDOW_CLOSING event to the container. WindowEvent windowClosingEvent = new WindowEvent(dialog, WindowEvent.WINDOW_CLOSING); dialog.dispatchEvent(windowClosingEvent); // Invoke garbage collection. Runtime.getRuntime().gc();
By following this approach, top-level containers can be removed at runtime while minimizing memory leaks and the risk of OutOfMemoryErrors.
The above is the detailed content of How to Effectively Remove Top-Level Containers in Swing to Prevent Memory Leaks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


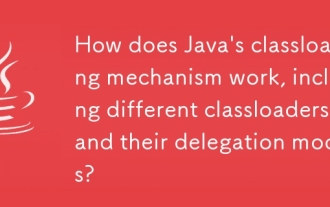
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
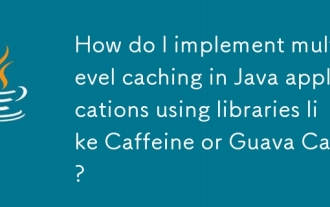
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
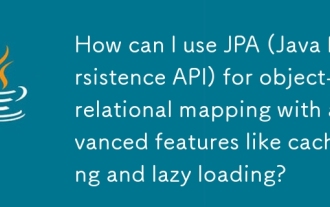
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
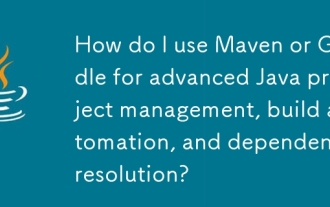
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
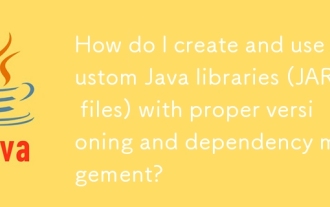
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
