JavaScript `apply()` vs. `call()`: When Should I Use Which?
Dec 22, 2024 pm 07:07 PMInvocation Methods in JavaScript: Exploring apply() vs. call()
When invoking functions in JavaScript, you have two primary methods at your disposal: apply() and call(). Both allow you to execute functions with a specified this value, but they differ in the way arguments are passed.
apply(thisValue, argumentsArray)
- Constructs an array from the provided argumentsArray.
- Invokes the function with the specified thisValue and the elements of the array as individual arguments.
- Example:
const func = function() { console.log("Hello world!"); }; func.apply(null, ["John", 35]); // Invokes "Hello world!" and logs "John" and 35.
call(thisValue, arg1, arg2, ...argN)
- Treats the arguments as individual parameters.
- Invokes the function with the specified thisValue and the arguments provided as separate values.
- Example:
func.call(null, "John", 35); // Invokes "Hello world!" and logs "John" and 35.
Mnemonics:
- Apply: A for array of arguments.
- Call: C for comma-separated arguments.
Performance Differences:
- There are generally negligible performance differences between apply() and call().
- However, apply() may be slightly faster when dealing with a large number of arguments.
Best Use Cases:
- Use apply(): When you have an array of arguments that you want to pass to a function, especially when the number of arguments is unknown in advance.
- Use call(): When you want to pass individual arguments to a function and have more control over their ordering.
ES6 Additions:
- In ES6, you can use the spread operator with call() to spread an array into individual arguments.
- Example:
func.call(null, ...["John", 35]); // Same as func.apply(null, ["John", 35]).
The above is the detailed content of JavaScript `apply()` vs. `call()`: When Should I Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
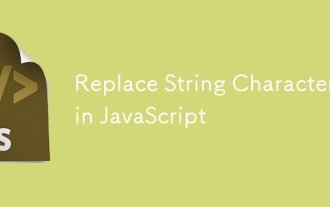
Replace String Characters in JavaScript
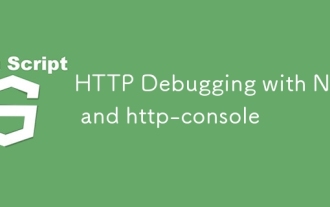
HTTP Debugging with Node and http-console
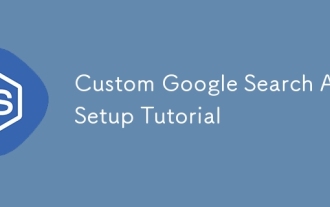
Custom Google Search API Setup Tutorial
