How can I store multiple values under a single key in a Java HashMap?
Storing Multiple Values under a Single Key in a HashMap
Maps in Java associate keys with single values, but you may encounter the need to store multiple values under the same key. This question explores how to achieve this functionality.
Map with List as Value
One approach is to use a map where the value is a list. This allows you to store multiple values as a list associated with the key. However, this has the limitation that the list can contain any number of values, not just two.
Using a Wrapper Class
You could also create a separate wrapper class that holds the values and place instances of this wrapper in the map. This approach requires defining a class and creating instances for each key, resulting in additional code.
Tuple-Based approach
If you want to enforce a fixed number of values, you can use a tuple class (often found in libraries or by implementing your own). Maps can then store tuples as values, ensuring a consistent structure for multiple values under a key.
Multiple Maps
Another option is to use multiple maps, one for each value. This approach is straightforward but may introduce the risk of the maps getting out of sync if not handled carefully.
Examples
Map with List as Value
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
Using a Wrapper Class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
|
Tuple-Based Approach
This approach requires a tuple class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
|
Multiple Maps
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
The above is the detailed content of How can I store multiple values under a single key in a Java HashMap?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
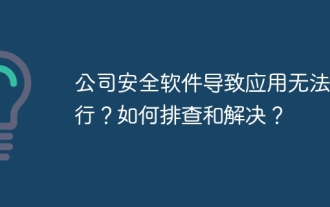
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
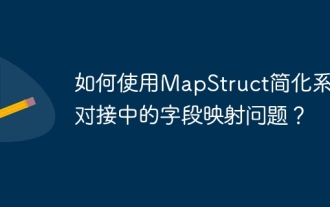
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
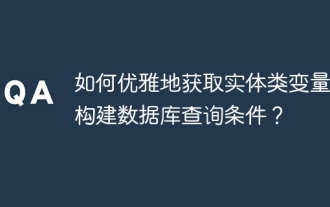
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
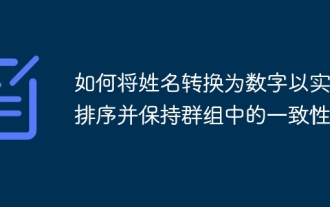
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
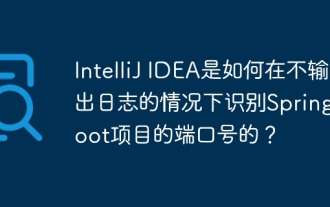
Start Spring using IntelliJIDEAUltimate version...
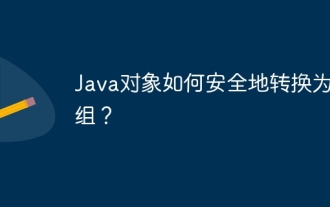
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
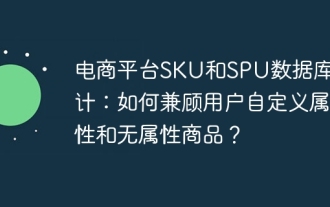
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
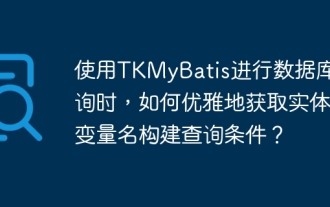
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
