


How Can I Efficiently Extract href Attributes from HTML Using the DOM API?
Grabbing the href Attribute: A DOM-Based Solution
When seeking to extract the href attributes from HTML, regex expressions may encounter limitations. For scenarios where the href attribute is not placed first in the tag, a more reliable approach is to utilize the DOM API.
Using DOM to Grab href Attributes
Consider the following PHP code:
$dom = new DOMDocument; $dom->loadHTML($html); foreach ($dom->getElementsByTagName('a') as $node) { echo $dom->saveHtml($node), PHP_EOL; }
This code loads the HTML content into a DOMDocument object, iterates through all elements using getElementsByTagName, and outputs the outerHTML of each element.
Accessing Node Values and Attributes
To extract specific information from the DOM nodes, you can use the following methods:
- nodeValue: Returns the text value of the node.
- hasAttribute('href'): Checks if the href attribute exists.
- getAttribute('href'): Retrieves the value of the href attribute.
- setAttribute('href', 'new value'): Changes the href attribute to a new value.
- removeAttribute('href'): Removes the href attribute from the node.
XPath for Attribute Querying
XPath can also be used to directly query for href attributes:
$xpath = new DOMXPath($dom); $nodes = $xpath->query('//a/@href'); foreach($nodes as $href) { echo $href->nodeValue; // Echo current attribute value $href->nodeValue = 'new value'; // Set new attribute value $href->parentNode->removeAttribute('href'); // Remove attribute }
By leveraging the capabilities of the DOM API, you can efficiently parse HTML content and manipulate a tags, including extracting and modifying their href attributes.
The above is the detailed content of How Can I Efficiently Extract href Attributes from HTML Using the DOM API?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
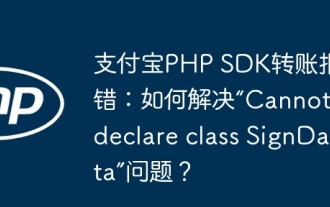
Alipay PHP...
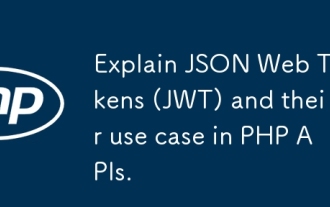
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
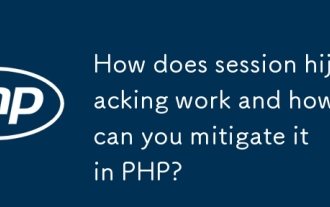
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
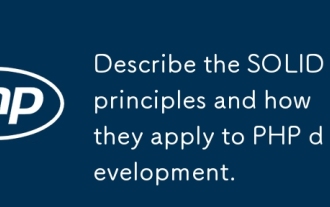
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
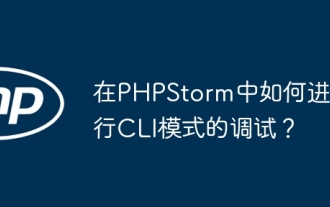
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
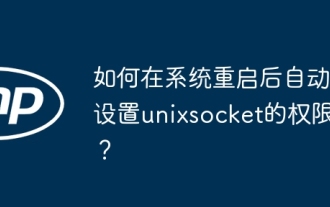
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
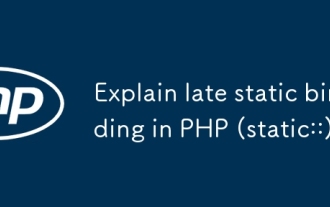
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
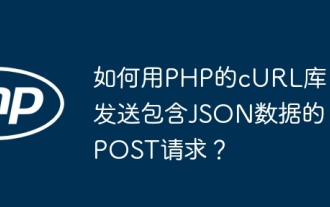
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
