How Can jQuery.animate() Be Used for Cross-Browser CSS Rotation?
Cross-Browser CSS Rotation with jQuery Animate()
Introduction
CSS rotation requires cross-browser compatibility to work effectively. This article aims to tackle this challenge by exploring issues and providing solutions for achieving rotation using jQuery.animate().
jQuery Rotate Issue
The provided code snippet demonstrates how CSS transforms are incompatible with jQuery.animate(). While css() can successfully rotate elements, animate() fails to do so.
Solution
The solution involves utilizing the step callback within the animation. This callback provides access to the current animation position, allowing us to manually apply the rotation transform with CSS. Here's the modified code:
function AnimateRotate(angle) { // Get the jQuery object for better performance var $elem = $('#MyDiv2'); // Use a pseudo object for animation, starting from `0` to `angle` $({ deg: 0 }).animate({ deg: angle }, { duration: 2000, step: function(now) { // Set the rotation using the CSS transform property $elem.css({ transform: 'rotate(' + now + 'deg)' }); } }); }
jQuery Plugin for Convenience
To simplify usage, a jQuery plugin can be created:
$.fn.animateRotate = function(angle, duration, easing, complete) { return this.each(function() { var $elem = $(this); $({ deg: 0 }).animate({ deg: angle }, { duration: duration, easing: easing, step: function(now) { $elem.css({ transform: 'rotate(' + now + 'deg)' }); }, complete: complete || $.noop }); }); };
Advanced Features
Optimized Order of Arguments:
The plugin's updated version supports an optimized order for arguments: angle, duration, easing, complete.
Toggle Functionality:
For scenarios requiring toggling rotation back and forth, a start parameter can be added to the function, allowing for more flexibility.
Conclusion:
The provided solutions effectively resolve the issue of rotating elements with jQuery.animate(). By leveraging the step callback and creating a jQuery plugin, developers can easily achieve cross-browser compatibility for CSS rotation.
The above is the detailed content of How Can jQuery.animate() Be Used for Cross-Browser CSS Rotation?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










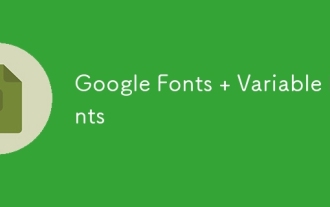
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
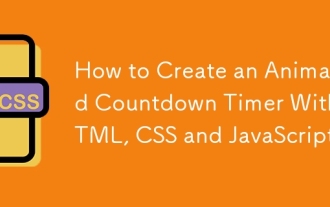
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
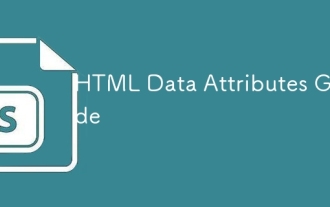
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
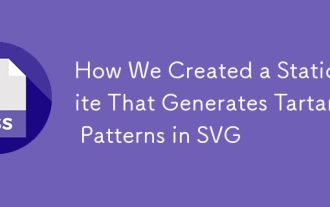
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
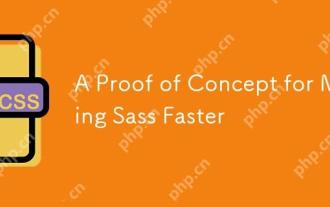
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
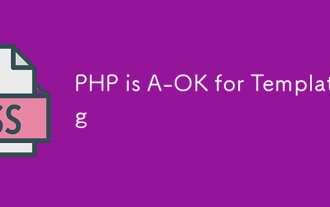
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
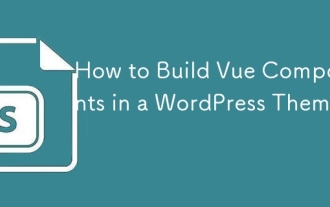
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
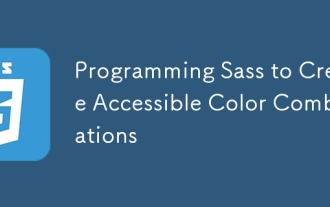
We are always looking to make the web more accessible. Color contrast is just math, so Sass can help cover edge cases that designers might have missed.
