How Can Selenium Access and Interact with Elements Hidden within Shadow DOM?
Shadow Root and Selenium: Navigating the Browsing Data Popup
Accessing elements within the #shadow-root (open) while clearing browsing data in Chrome using Selenium can present challenges. To overcome this, we must understand Shadow DOM and Selenium's capabilities.
Shadow DOM
Shadow DOM allows web developers to encapsulate HTML and style within custom components, creating isolated and independent UI elements. These components, termed "shadow hosts," have their own shadow tree, a hidden DOM fragment.
Selenium and Shadow DOM
Selenium doesn't natively support Shadow DOM elements as they lie outside the main DOM tree. To interact with these elements, we must first access their shadow tree.
Traversing Shadow DOM
1. Identifying the Shadow Host:
Locate the shadow host in the main DOM using Selenium's findElement method.
2. Extracting Shadow Root:
Use our custom method getShadowRoot to access the shadow root associated with the shadow host.
3. Navigating Shadow Tree:
Traverse the shadow tree by using our getShadowElement method, passing in the shadow root and CSS selector of the target element.
Example: Clearing Browsing Data
Using our custom methods, we can navigate the multi-level Shadow DOM to access and click the Clear Browsing Data button:
WebElement shadowHostL1 = driver.findElement(By.cssSelector("settings-ui")); WebElement shadowElementL1 = getShadowElement(driver, shadowHostL1, "settings-main"); WebElement shadowElementL2 = getShadowElement(driver, shadowElementL1, "settings-basic-page"); WebElement shadowElementL3 = getShadowElement(driver, shadowElementL2, "settings-section > settings-privacy-page"); WebElement shadowElementL4 = getShadowElement(driver, shadowElementL3, "settings-clear-browsing-data-dialog"); WebElement shadowElementL5 = getShadowElement(driver, shadowElementL4, "#clearBrowsingDataDialog"); WebElement clearData = shadowElementL5.findElement(By.cssSelector("#clearBrowsingDataConfirm")); clearData.click();
Alternatively, we can use a single JavaScript call:
WebElement clearData = (WebElement) js.executeScript("return document.querySelector(...[CSS selector to traverse Shadow DOM]...");
The above is the detailed content of How Can Selenium Access and Interact with Elements Hidden within Shadow DOM?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










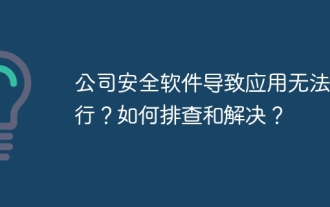
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
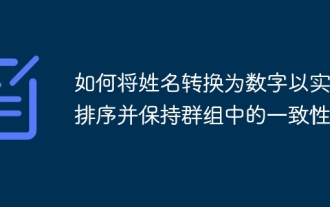
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
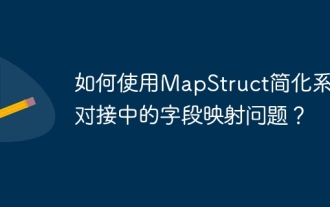
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
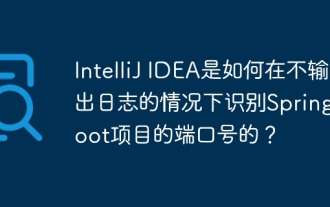
Start Spring using IntelliJIDEAUltimate version...
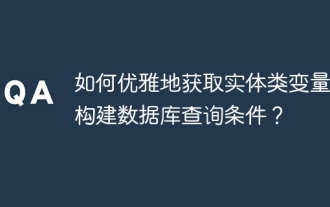
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
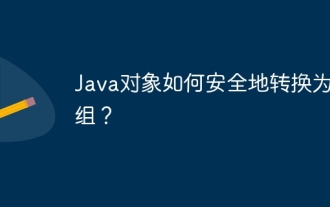
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
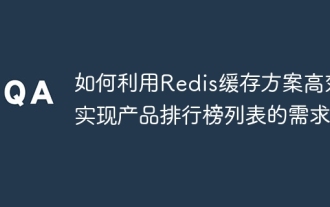
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
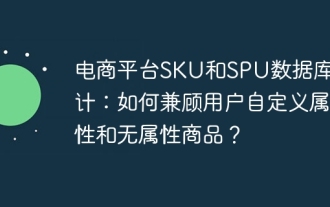
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
