How Can I Clone Go Structs with Unexported Fields?
Struct Cloning with Unexported Fields
In Go, structs with unexported fields present a challenge for cloning objects. Consider a type defined as:
type T struct { S string is []int }
A simple assignment like below will not create an independent copy:
p := T{"some string", []int{10, 20}} q := p
This is because the type's unexported field (is) is inaccessible and cannot be copied over explicitly.
Cloning via Custom Method
One workaround is to provide a Clone method within the package owning the type. However, this only works for types within the same package.
Limitations of Third-Party Types
If a type with unexported fields resides in a third-party package, there is no direct way to clone it. This is by design, as unexported fields should remain private to the declaring package.
Alternative Approach
While it's not possible to clone unexported fields, it is possible to create new structs with empty (zero) values for those fields:
var r somepackage.T s := somepackage.T{S: p.S}
Unsafe Practices
Using the unsafe package is not recommended for this purpose, as it can lead to unexpected and potentially unsafe behavior.
Copying Unexported Fields
When assigning one struct to another of the same type, unexported fields are properly copied. However, modifying those fields is not possible (they can only be nil or the same pointer value as the original).
type person struct { Name string age *int } age := 22 p := &person{"Bob", &age} p2 := new(person) *p2 = *p // Copy unexported field fmt.Println(p2) // Outputs: &{Bob 0x414020}
The above is the detailed content of How Can I Clone Go Structs with Unexported Fields?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
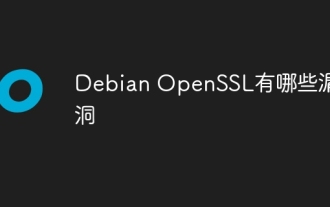
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
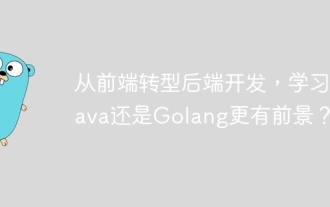
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
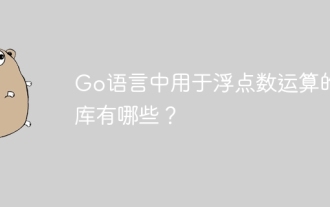
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
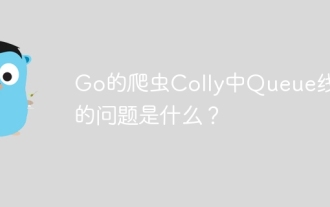
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
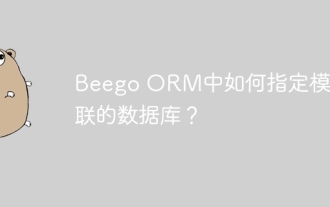
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
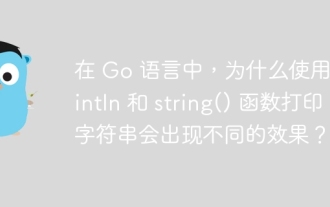
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
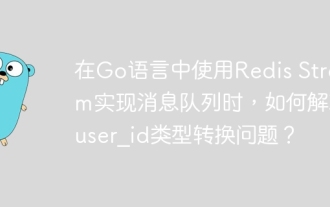
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
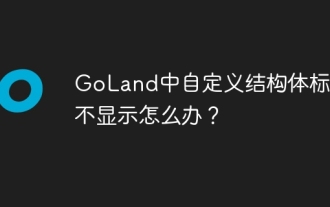
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
