How Can I Truncate Strings in PHP to the Nearest Word?
Truncating Strings in PHP to the Nearest Word
To crop a string at the end of the last word before a specified character count, PHP provides several methods.
Using wordwrap Function:
The wordwrap function divides text into multiple lines with a maximum width, automatically breaking at word boundaries. By taking only the first line, you can truncate the text to the nearest word:
$truncated_string = substr($string, 0, strpos(wordwrap($string, $desired_width), "\n"));
Edge Case Handling:
This method doesn't handle texts shorter than the desired width. For that, use the following:
if (strlen($string) > $desired_width) { $string = substr($string, 0, strpos(wordwrap($string, $desired_width), "\n")); }
Token Truncation:
To resolve potential issues with newlines in the text, this method splits the text into tokens (words, whitespace, and newlines) and accumulates their lengths:
function tokenTruncate($string, $desired_width) { $parts = preg_split('/([\s\n\r]+)/', $string, null, PREG_SPLIT_DELIM_CAPTURE); $length = 0; $last_part = 0; for (; $last_part < count($parts); ++$last_part) { $length += strlen($parts[$last_part]); if ($length > $desired_width) { break; } } return implode(array_slice($parts, 0, $last_part)); }
This method also handles UTF8 characters.
Unit Testing:
class TokenTruncateTest extends PHPUnit_Framework_TestCase { // ... test cases ... }
Additional Notes:
- This method relies on word boundaries.
- Handling special characters like emojis or different languages may require additional considerations.
The above is the detailed content of How Can I Truncate Strings in PHP to the Nearest Word?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
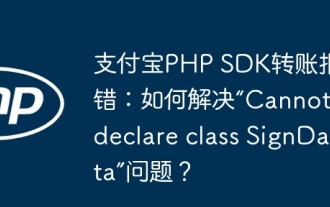
Alipay PHP...
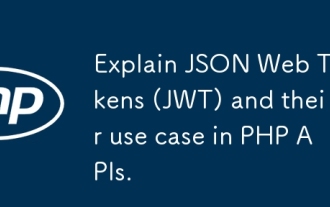
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
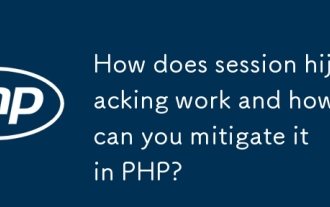
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
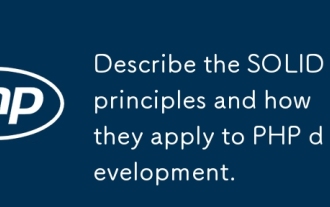
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
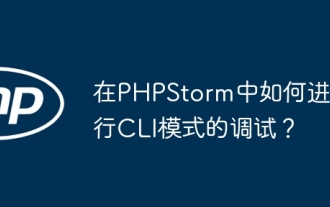
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
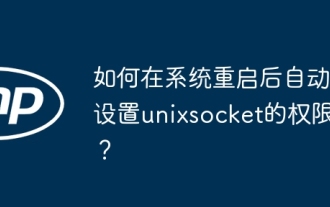
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
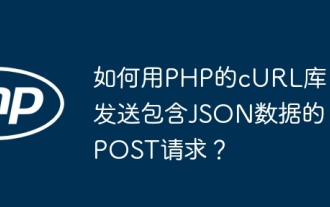
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
