


How Can Promise.all Improve Concurrent Async Operation Handling and Avoid Unhandled Rejections?
Waiting for Concurrent Async Operations
Parallel Execution with Promise.all
To trigger multiple async operations concurrently, avoid using the pattern where you acquire promises and then await them sequentially. Instead, leverage the Promise.all function:
const [value1, value2] = await Promise.all([getValue1Async(), getValue2Async()]);
This approach initiates both async operations simultaneously and provides the results in an array.
Handling Rejected Promises
The provided solution runs the operations in parallel, but waits for the first to complete before waiting for the second. While this allows parallel execution, it introduces issues in handling rejected promises.
If the first promise takes longer to complete and the second fails, Promise.all will fail immediately, preventing the first promise from rejecting. This can result in an unhandled rejection error.
Syntax for Parallel Await
Currently, there isn't a designated await syntax for parallel waiting, hence the alternative of using Promise.all. Discussions have emerged regarding await.all, indicating the possibility of future enhancements.
Comparison of Approaches
Consider the following example, where getValue1Async takes 500ms to resolve and getValue2Async takes 100ms to reject:
Sequential Execution:
async () => { try { const value1 = await getValue1Async(); const value2 = await getValue2Async(); } catch (e) { console.error(e); } };
Concurrent Execution with Promise.all:
async () => { try { const [value1, value2] = await Promise.all([getValue1Async(), getValue2Async()]); } catch (e) { console.timeEnd("Promise.all", e); } };
Output:
- Sequential: Console output shows a delay of 500ms before the error is logged.
- Concurrent: Console output shows an error log after 100ms.
Conclusion:
Using Promise.all effectively enables parallel execution of async operations and ensures proper handling of rejected promises.
The above is the detailed content of How Can Promise.all Improve Concurrent Async Operation Handling and Avoid Unhandled Rejections?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










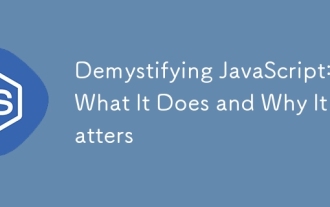
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
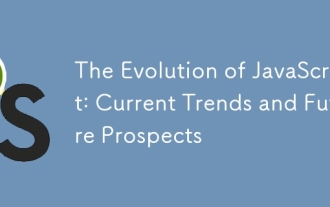
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
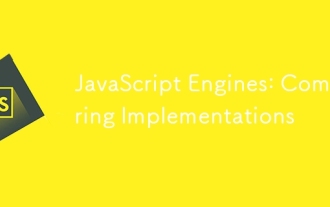
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
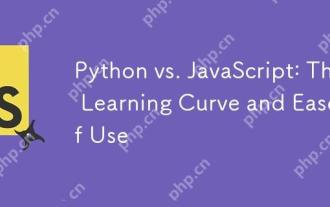
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
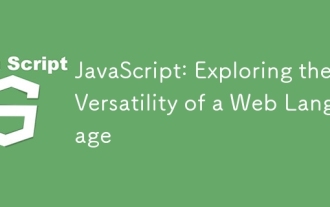
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
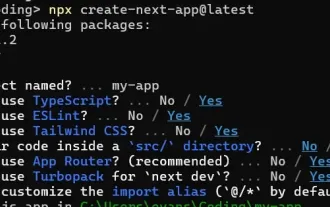
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
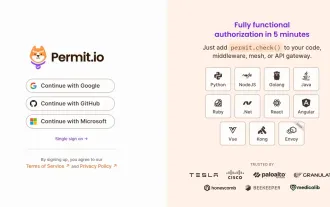
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
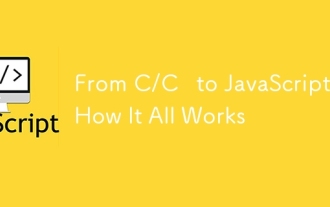
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
