How Can I Use ANTLR to Parse and Evaluate Simple Mathematical Expressions?
Certainly, here's the answer for the given title and content:
ANTLR: A Simple Step-by-Step Guide with Example
ANTLR, or ANother Tool for Language Recognition, is a powerful parser generator tool that allows you to define grammars and generate parsers in various programming languages. If you're a beginner, you may find the ANTlr.org examples a bit overwhelming. This guide provides a simplified example to help you understand how ANTLR works.
Creating the Grammar File
To start, define a simple grammar in a text file, e.g., "Exp.g". The following grammar lets you evaluate basic mathematical expressions with four operators ( , -, *, /) and parentheses:
grammar Exp; eval : additionExp EOF ; additionExp : multiplyExp ( '+' multiplyExp | '-' multiplyExp )* ; multiplyExp : atomExp ( '*' atomExp | '/' atomExp )* ; atomExp : Number | '(' additionExp ')' ; Number : ('0'..'9')+ ('.' ('0'..'9')+)? ;
Generating the Lexer and Parser
Assuming you have the ANTLR jar downloaded, run the following command to generate the lexer (ExpLexer.java) and parser (ExpParser.java) classes:
java -cp antlr-3.2.jar org.antlr.Tool Exp.g
Creating the Test Class
Now, create a test class, e.g., ANTLRDemo.java, that invokes the parser and processes the expression:
import org.antlr.runtime.*; public class ANTLRDemo { public static void main(String[] args) throws Exception { ANTLRStringStream in = new ANTLRStringStream("12*(5-6)"); ExpLexer lexer = new ExpLexer(in); CommonTokenStream tokens = new CommonTokenStream(lexer); ExpParser parser = new ExpParser(tokens); System.out.println(parser.eval()); } }
Enhancing the Grammar with Java Code
To perform calculations, add Java code blocks within your grammar rules, enclosed in { }. Here's the modified grammar:
grammar Exp; eval : exp=additionExp {$value = $exp.value;} ; additionExp : m1=multiplyExp {$value = $m1.value;} ( '+' m2=multiplyExp {$value += $m2.value;} | '-' m2=multiplyExp {$value -= $m2.value;} )* ; // ...
Running the Example
Compile all generated and test classes, then run the ANTLRDemo class:
// *nix/MacOS java -cp antlr-3.2.jar org.antlr.Tool Exp.g // 1 javac -cp .:antlr-3.2.jar ANTLRDemo.java // 2 java -cp .:antlr-3.2.jar ANTLRDemo // 3 // Windows java -cp antlr-3.2.jar org.antlr.Tool Exp.g // 1 javac -cp .;antlr-3.2.jar ANTLRDemo.java // 2 java -cp .;antlr-3.2.jar ANTLRDemo // 3
This will calculate and display the result of "12*(5-6)" to your console.
This simple example demonstrates the basics of ANTLR. To learn more, explore the ANTLR wiki, tutorials, and documentation.
The above is the detailed content of How Can I Use ANTLR to Parse and Evaluate Simple Mathematical Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
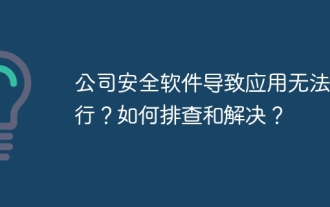
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
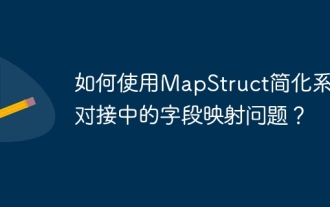
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
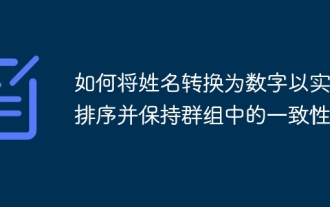
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
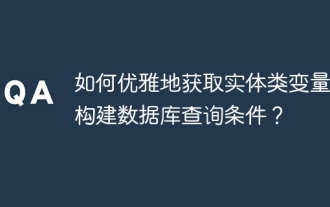
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
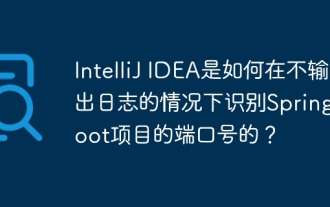
Start Spring using IntelliJIDEAUltimate version...
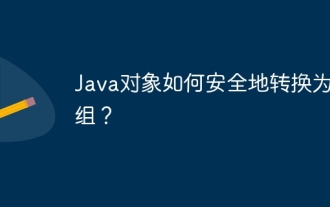
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
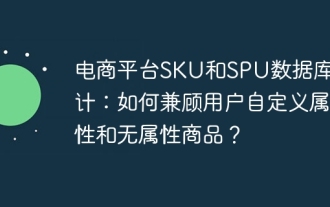
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
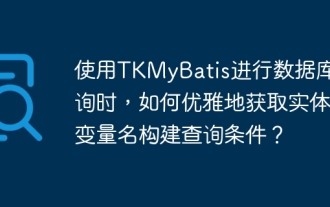
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
