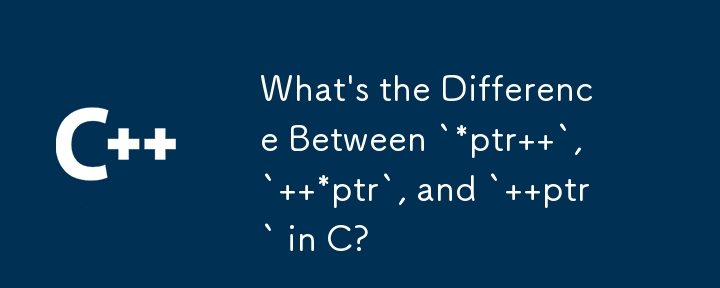
Understanding Pointer Expressions: ptr , ptr, and *ptr
In C programming, these three expressions are commonly used to manipulate pointers and the values they point to. Understanding their behavior is crucial for effectively working with pointers.
1. *ptr
- This expression effectively dereferences the pointer first, returning the value it points to.
-
Then, it increments the pointer by one unit, pointing it to the next element in the array or the next character in the string.
- For example, if ptr points to the first element of an array, *ptr will return the value of that element and then increment ptr to point to the second element.
2. * ptr
- Unlike the previous expression, this one first increments the pointer by one unit.
-
Then, it dereferences the pointer, returning the value the pointer now points to.
- This is useful when you need to access the value of the next element without dereferencing the current pointer.
3. *ptr
-
This expression directly increments the value at the location pointed to by ptr.
- It first dereferences the pointer, returning the value it points to.
-
Then, it increments the value by one unit.
- This can be used to modify the value pointed to by ptr.
Examples:
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = arr;
printf("%d ", *ptr++); // Prints 1 and increments ptr to the second element
printf("%d ", *++ptr); // Increments ptr to the third element and prints 3
printf("%d\n", ++*ptr); // Increments the value at the third element and prints 4
Copy after login
Output:
The above is the detailed content of What's the Difference Between `*ptr `, ` *ptr`, and ` ptr` in C?. For more information, please follow other related articles on the PHP Chinese website!