How Can Java Applications Send Emails via Gmail, Yahoo, or Hotmail?
Sending Emails Using GMail, Yahoo, or Hotmail in Java Applications
Question:
Can Java applications utilize email accounts like GMail, Yahoo, or Hotmail to send emails? How can this be achieved?
Answer:
Step 1: Import JavaMail API
Before proceeding, ensure that you have downloaded and added the required JavaMail API jars to your classpath.
Step 2: GMail Configuration
The following Java code snippet demonstrates sending an email using a GMail account:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
|
Note: Replace the USER_NAME, PASSWORD, RECIPIENT, subject, and body variables with the appropriate values.
Additional Considerations:
- Proper error handling in the catch blocks should be implemented.
- You may need to adjust the code for different email providers by updating the server address (host) and port (e.g., for Yahoo: host="smtp.mail.yahoo.com" and port="587").
The above is the detailed content of How Can Java Applications Send Emails via Gmail, Yahoo, or Hotmail?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
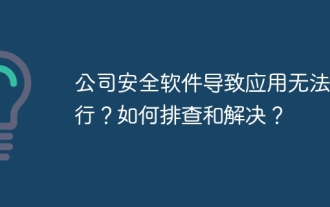
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
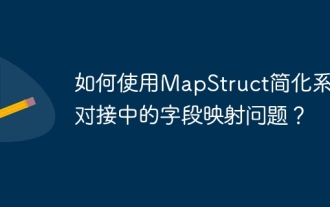
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
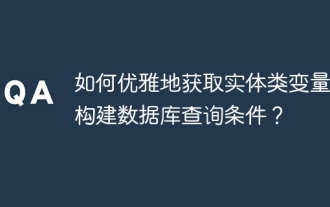
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
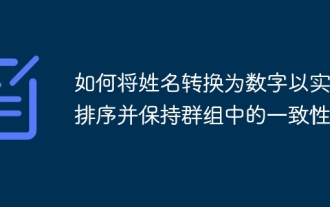
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
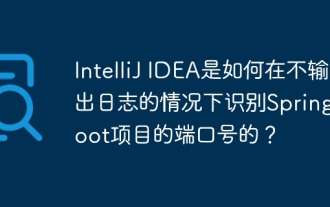
Start Spring using IntelliJIDEAUltimate version...
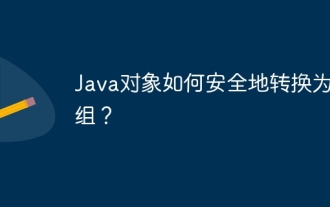
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
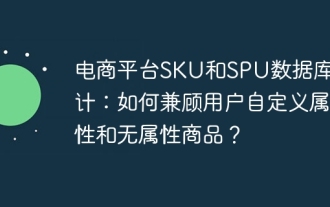
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
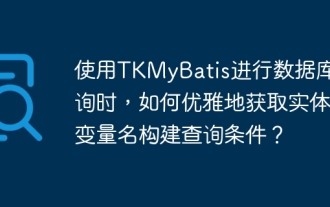
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
