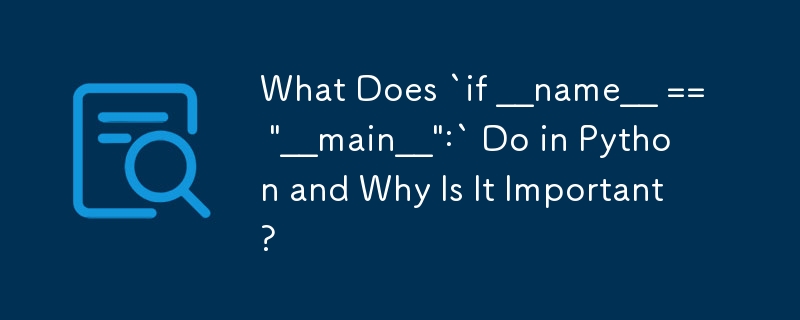
What Does name == "__main__": Do and Why Is It Necessary?
When a Python script is executed as the main program, the interpreter assigns the special variable __name__ to "__main__". If the script is imported as a module by another program, __name__ is instead set to the name of the importing module.
To understand why this is important, consider the following example:
# Suppose this is foo.py.
print("before import")
import math
print("before function_a")
def function_a():
print("Function A")
print("before function_b")
def function_b():
print("Function B {}".format(math.sqrt(100)))
print("before __name__ guard")
if __name__ == '__main__':
function_a()
function_b()
print("after __name__ guard")
Copy after login
Execution Flow:
-
Special Variables Setup:
-
name is set to "__main__" since foo.py is run as the main program.
-
Code Execution:
Importance of name Check:
This check is crucial because:
-
Protection from Accidental Invocation: If foo.py doesn't have the name check, importing it as a module would trigger the script to run using the importing script's command line arguments, which is usually undesired.
-
Pickle File Issue: If foo.py has custom classes saved to a pickle file, unpickling it in another script would import foo.py and execute its code without the name check, leading to the above issues.
Additional Notes:
- You can have multiple name checks in a script, but it's uncommon.
- Running the script foo2.py with the name checks included results in "a1", "m1", "a2", "b", "a3", "m2", "t2", while removing the name check results in "a1", "a2", "b", "a3", "t2".
- Running foo3.py as a script results in "t1", "a1", "a2", "b", "a3", "t2", while importing it as a module results in nothing being executed.
- Setting __name__ to "__main__" in foo4.py (even when imported as a module) means the script will always run when imported, as __name__ == __main__ will always evaluate to True.
The above is the detailed content of What Does `if __name__ == '__main__':` Do in Python and Why Is It Important?. For more information, please follow other related articles on the PHP Chinese website!