Is Pointer Assignment Atomic in Go, and How Can It Be Made Safe?
Assigning Pointers Atomically in Go
Go provides a variety of tools for managing concurrency, but one question that often arises is whether assigning a pointer is an atomic operation.
Atomic Operations in Go
In Go, the only operations that are guaranteed to be atomic are those found in the sync.atomic package. As such, assigning a pointer is not natively atomic.
Safe Pointer Assignment
To assign a pointer atomically, you have two options:
- Acquire a Lock: Using a lock (e.g., sync.Mutex) ensures that only one goroutine can access the pointer at a time, preventing race conditions.
- Use Atomic Primitives: The sync.atomic package offers atomic primitives like atomic.StorePointer and atomic.LoadPointer, which can be used to atomically assign and retrieve pointer values.
Example with Mutex
Here's an example using a sync.Mutex to protect a pointer assignment:
import "sync" var secretPointer *int var pointerLock sync.Mutex func CurrentPointer() *int { pointerLock.Lock() defer pointerLock.Unlock() return secretPointer } func SetPointer(p *int) { pointerLock.Lock() secretPointer = p pointerLock.Unlock() }
Example with Atomic Primitives
Alternatively, you can use atomic primitives to achieve atomicity:
import "sync/atomic" import "unsafe" type Struct struct { p unsafe.Pointer // some pointer } func main() { data := 1 info := Struct{p: unsafe.Pointer(&data)} fmt.Printf("info is %d\n", *(*int)(info.p)) otherData := 2 atomic.StorePointer(&info.p, unsafe.Pointer(&otherData)) fmt.Printf("info is %d\n", *(*int)(info.p)) }
Considerations
- Using sync.atomic can complicate code due to the need to cast to unsafe.Pointer.
- Locks are generally considered idiomatic in Go, while using atomic primitives is discouraged.
- An alternative approach is to use a single goroutine for pointer access and communicate commands via channels, which aligns better with Go's concurrency model.
The above is the detailed content of Is Pointer Assignment Atomic in Go, and How Can It Be Made Safe?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










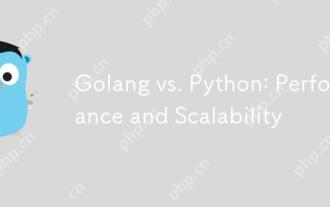
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
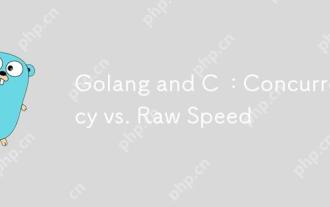
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
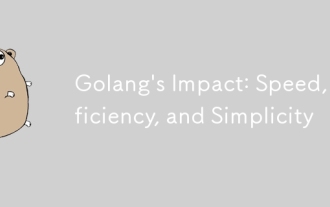
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
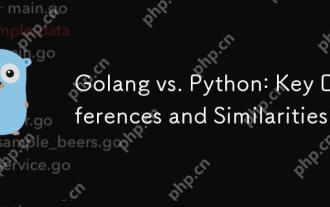
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
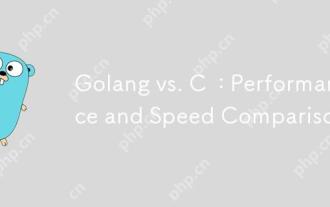
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
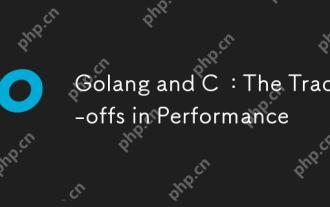
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
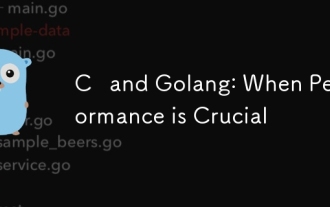
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
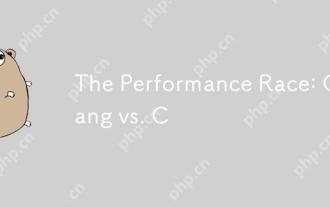
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
