How Can I Use Custom Thread Pools with Java 8 Parallel Streams?
Customizable Thread Pools in Java 8 Parallel Streams
Controlling thread resource allocation within parallel streams enhances application performance and modularity. However, it remains unclear how to designate custom thread pools for specific stream operations in Java 8.
This article explores the issue and provides a solution, overcoming the limitation of shared thread pools in parallel streams.
Problem Statement
Consider a server application utilizing parallel streams. To prevent sluggish tasks within one module from impacting others, thread pool compartmentalization is desired. However, the standard parallel stream implementation employs a shared thread pool for all operations.
As exemplified by the following code snippet, a compromised task delays other threads indefinitely:
// CPU-intensive tasks in separate threads leveraging parallel streams ExecutorService es = Executors.newCachedThreadPool(); es.execute(() -> runTask(1000)); // Incorrect task es.execute(() -> runTask(0)); es.execute(() -> runTask(0)); es.execute(() -> runTask(0)); es.execute(() -> runTask(0)); es.execute(() -> runTask(0));
Solution
To execute parallel operations within a designated fork-join pool, employ it as a task within the pool. This isolates the operation from the shared pool:
final int parallelism = 4; ForkJoinPool forkJoinPool = null; try { forkJoinPool = new ForkJoinPool(parallelism); final List<Integer> primes = forkJoinPool.submit(() -> { // Parallel task here, for example: return IntStream.range(1, 1_000_000).parallel() .filter(PrimesPrint::isPrime) .boxed() .collect(Collectors.toList()); }).get(); System.out.println(primes); } catch (Exception e) { throw new RuntimeException(e); } finally { if (forkJoinPool != null) { forkJoinPool.shutdown(); } }
This technique leverages ForkJoinTask.fork()'s behavior: "Asynchronously execute this task in the pool the current task is running in, if applicable, or using the ForkJoinPool.commonPool() if not in ForkJoinPool()."
The above is the detailed content of How Can I Use Custom Thread Pools with Java 8 Parallel Streams?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
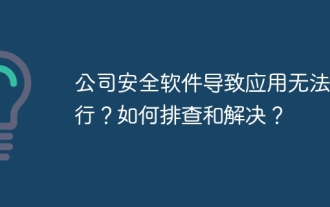
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
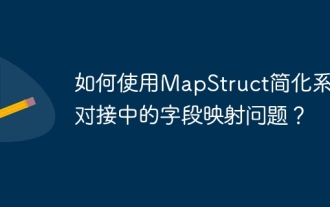
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
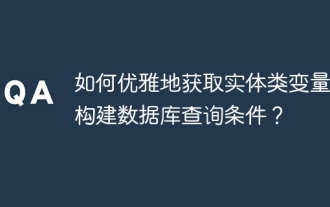
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
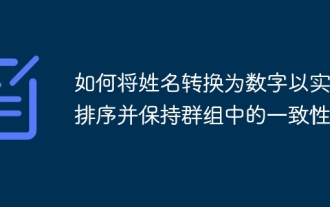
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
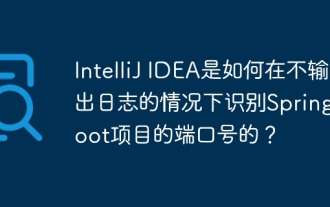
Start Spring using IntelliJIDEAUltimate version...
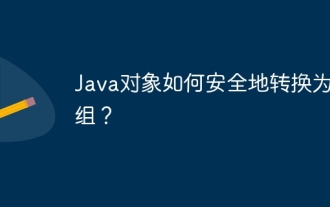
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
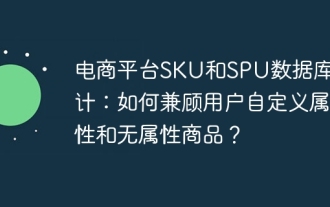
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
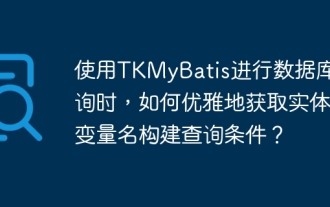
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
