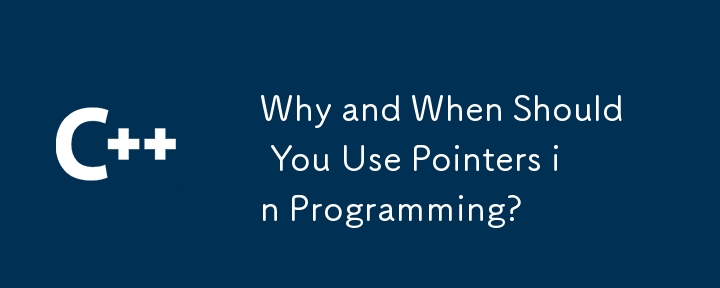
Why Use Pointers?
Basic Considerations
Pointers are a fundamental concept in programming that grant the ability to manipulate memory and variable references. While normal variables store the actual values, pointers store the addresses of memory locations where the actual data resides.
Advantages of Pointers Over Normal Variables
-
Indirect Addressing: Pointers allow indirect access to data, enabling efficient manipulation of complex data structures like linked lists and trees.
-
Pass-by-Reference: In functions, pointers can pass variables as references rather than values, preventing unnecessary copying of large data structures.
-
Memory Efficiency: By pointing to data rather than storing it directly, pointers conserve memory, especially for recursive data structures where the same value may be needed in multiple places.
When and Where to Use Pointers
-
Data Structures: Pointers are essential for manipulating complex data structures, such as linked lists, trees, and graphs.
-
Dynamic Memory Allocation: Pointers are used to dynamically allocate memory using functions like malloc() and free().
-
Function Parameters: Functions can receive pointers as parameters, allowing them to modify the actual values passed to them.
-
Indirect Access: Pointers enable indirect access to variables, which is useful for accessing members of structs and arrays.
Pointers and Arrays
Similarities and Differences:
- Arrays and pointers are closely related.
- The array name in an array declaration serves as a constant pointer to the first element of the array.
- Arrays and pointers can be dereferenced using the * operator to access the elements they point to.
Example:
int myArray[] = {1, 2, 3};
int *ptr = myArray; // ptr points to the first element of myArray
printf("First element of array: %d\n", myArray[0]);
printf("First element of array via pointer: %d\n", *ptr);
// Iterate over the array using the pointer
for (size_t i = 0; i < 3; i++) {
printf("Element %zu: %d\n", i, ptr[i]);
}
Copy after login
The above is the detailed content of Why and When Should You Use Pointers in Programming?. For more information, please follow other related articles on the PHP Chinese website!