How to Read and Write Strings to Files in Android?
Read/Write a String from/to a File in Android
Reading and writing strings to and from files is a fundamental operation in Android development. This article will demonstrate how to accomplish these tasks using the operating system's built-in file I/O methods.
Writing a String to a File
To save a text string to an internal storage file, follow these steps:
- Create an OutputStreamWriter object using openFileOutput() and specify the file name and mode.
- Write the string to the OutputStreamWriter using write().
- Close the OutputStreamWriter.
Reading a String from a File
To retrieve the string from the saved file, follow these steps:
- Create an InputStream object using openFileInput().
- Create an InputStreamReader object using the InputStream.
- Create a BufferedReader object using the InputStreamReader.
- Read lines from the file using readLine().
- Append the lines to a StringBuilder object.
- Close the InputStream.
Example Code
Here's an example code snippet that demonstrates the aforementioned operations:
Writing:
private void writeToFile(String data, Context context) { try { OutputStreamWriter outputStreamWriter = new OutputStreamWriter(context.openFileOutput("config.txt", Context.MODE_PRIVATE)); outputStreamWriter.write(data); outputStreamWriter.close(); } catch (IOException e) { Log.e("Exception", "File write failed: " + e.toString()); } }
Reading:
private String readFromFile(Context context) { String ret = ""; try { InputStream inputStream = context.openFileInput("config.txt"); if (inputStream != null) { InputStreamReader inputStreamReader = new InputStreamReader(inputStream); BufferedReader bufferedReader = new BufferedReader(inputStreamReader); String receiveString = ""; StringBuilder stringBuilder = new StringBuilder(); while ((receiveString = bufferedReader.readLine()) != null) { stringBuilder.append("\n").append(receiveString); } inputStream.close(); ret = stringBuilder.toString(); } } catch (FileNotFoundException e) { Log.e("login activity", "File not found: " + e.toString()); } catch (IOException e) { Log.e("login activity", "Can not read file: " + e.toString()); } return ret; }
The above is the detailed content of How to Read and Write Strings to Files in Android?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










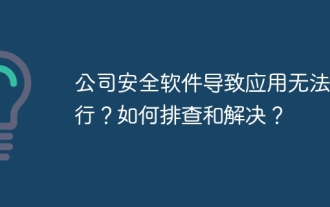
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
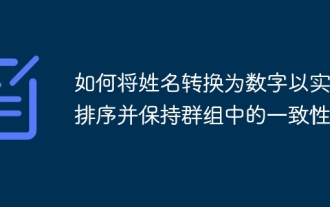
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
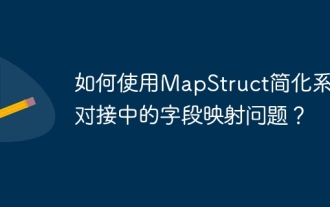
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
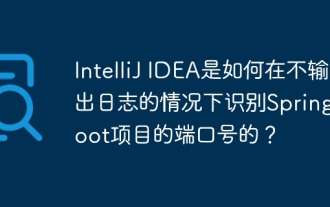
Start Spring using IntelliJIDEAUltimate version...
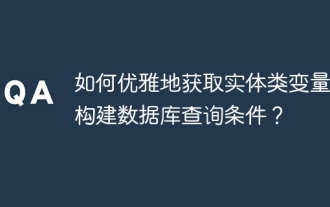
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
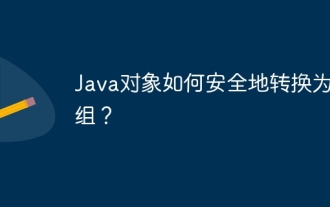
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
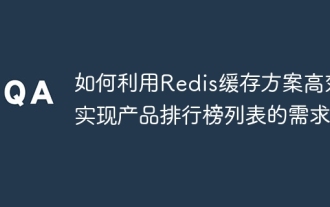
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
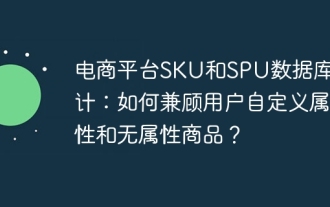
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
