Polymorphism in Java: How Does it Differ from Overriding and Overloading?
Polymorphism: Unveiling the Distinction from Overriding and Overloading
When it comes to polymorphism in Java, it's important to clarify the difference from overriding and overloading. Overloading refers to the existence of multiple methods with the same name but different parameter lists within the same class. Overriding, on the other hand, involves defining a method in a subclass that has the same signature (name and parameters) as a method in its superclass.
When considering polymorphism, the most accurate answer lies in the concept of abstract classes or interfaces. An abstract base class defines a method without an implementation, which is then implemented in its subclasses. This approach exemplifies polymorphism.
To illustrate, consider an abstract base class Human with an abstract method goPee(), which cannot be defined at the Human level but must be implemented in the subclasses Male and Female to accommodate gender-specific behavior.
public abstract class Human { public abstract void goPee(); }
public class Male extends Human { @Override public void goPee() { System.out.println("Stand Up"); } }
public class Female extends Human { @Override public void goPee() { System.out.println("Sit Down"); } }
In this example, the method goPee() is overridden in the subclasses to provide specific implementations. Polymorphism comes into play when we can tell a group of Humans to go pee, and they will perform the action according to their respective implementations.
public static void main(String[] args) { ArrayList<Human> group = new ArrayList<>(); group.add(new Male()); group.add(new Female()); // Tell Humans to go pee for (Human person : group) person.goPee(); }
Output:
Stand Up Sit Down
From this demonstration, it becomes clear that polymorphism is the ability for different objects within an inheritance hierarchy to exhibit different behaviors while responding to the same message. Overriding is the mechanism that enables this behavior, while overloading is a separate concept related to method signatures within the same class.
The above is the detailed content of Polymorphism in Java: How Does it Differ from Overriding and Overloading?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
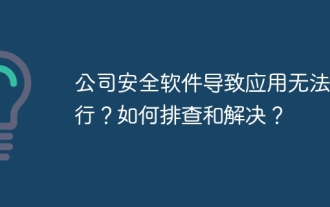
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
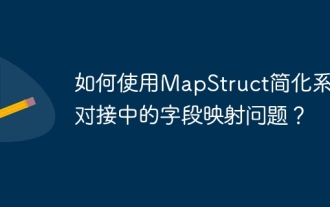
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
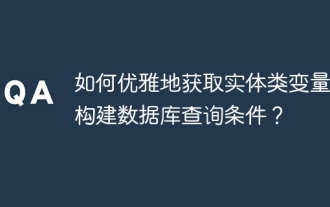
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
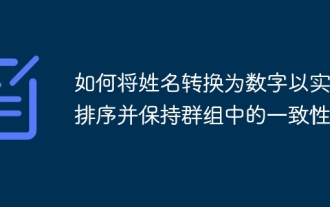
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
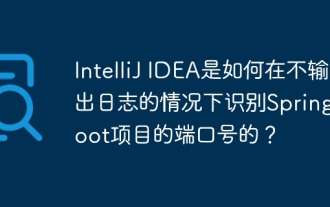
Start Spring using IntelliJIDEAUltimate version...
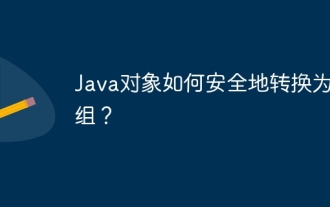
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
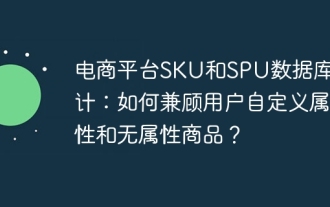
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
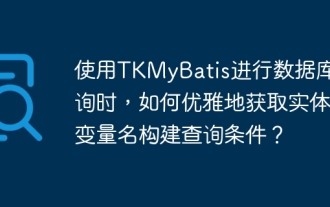
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
