


How Can JavaScript Detect HTML Element Content Overflow, Even with `overflow: visible`?
Checking Content Overflow in HTML Elements Using JavaScript
When an HTML element's content exceeds its defined dimensions, it can result in overflow. While visible overflow can be checked using standard checks, checking for overflow with the "overflow: visible" property can pose a challenge.
JavaScript Solution
To account for this, a JavaScript function can be employed:
function checkOverflow(el) { var curOverflow = el.style.overflow; // If overflow is 'visible' or not set if (!curOverflow || curOverflow === "visible") { // Temporarily set overflow to 'hidden' el.style.overflow = "hidden"; } var isOverflowing = el.clientWidth < el.scrollWidth || el.clientHeight < el.scrollHeight; // Restore original overflow style el.style.overflow = curOverflow; return isOverflowing; }
Usage:
Pass the HTML element in question as a parameter to the checkOverflow function to determine if its content is overflowing. The function will return true if there is overflow, otherwise it will return false.
Compatibility:
This solution has been tested in Firefox 3 and 40, Internet Explorer 6, and Chrome 0.2.149.30.
The above is the detailed content of How Can JavaScript Detect HTML Element Content Overflow, Even with `overflow: visible`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


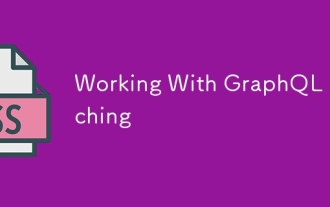
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
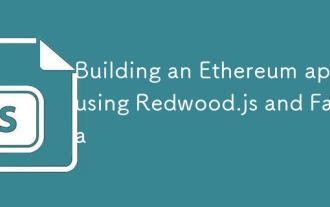
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
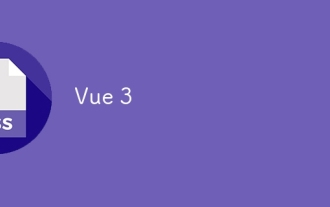
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
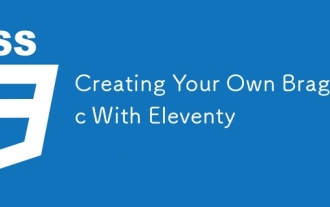
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
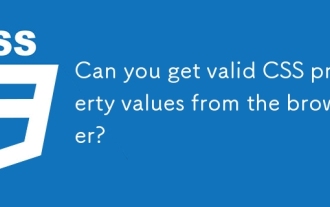
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
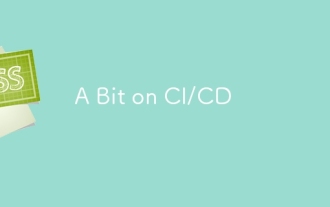
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
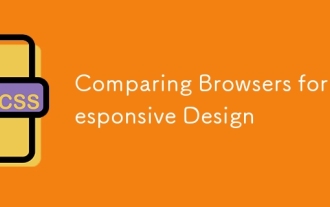
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
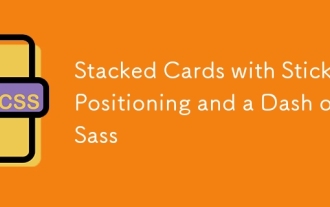
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
