


Why Does Comparing Custom Objects with `==` and `.equals()` Return `false` Even with Identical Field Values?
Comparing Objects with .equals() and == Operator
Question:
In a custom class with a string field, why do object comparisons using both the == operator and .equals() method return false, even when the field values are identical?
Answer:
The == operator compares object references, determining if the objects being compared are the same object in memory. On the other hand, .equals() compares the contents of objects.
In this case, using == results in false because object1 and object2 are not the same object in memory, even though they have the same field value. To determine if the objects are equal in terms of field values, .equals() should be used.
Revised equals() Method:
The equals() method in the provided code can be revised to compare the values of the a field:
1 2 3 4 5 6 7 |
|
Additional Note:
When overriding equals(), it is generally recommended to also override hashCode() to maintain the contract that equal objects have equal hash codes.
The above is the detailed content of Why Does Comparing Custom Objects with `==` and `.equals()` Return `false` Even with Identical Field Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
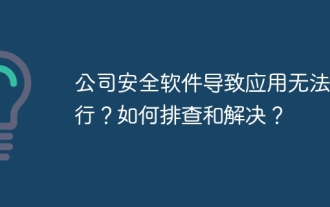
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
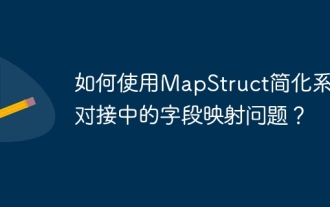
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
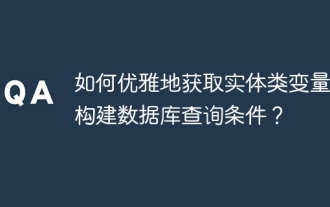
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
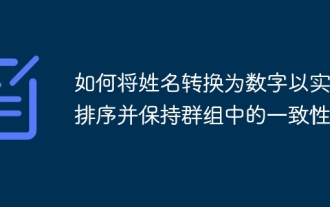
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
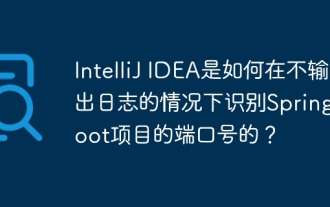
Start Spring using IntelliJIDEAUltimate version...
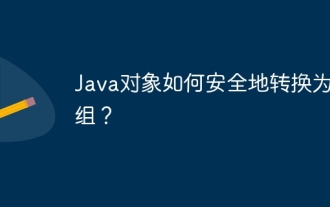
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
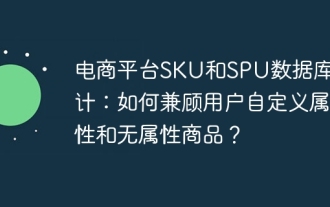
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
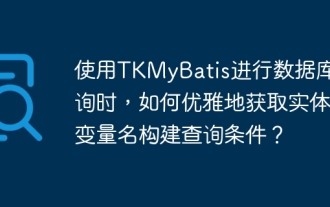
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
